用c++写一个五子棋的游戏
时间: 2023-12-03 20:02:57 浏览: 24
好的,以下是一个简单的五子棋游戏的C++代码,希望能帮到你:
```
#include <iostream>
#include <cstring>
using namespace std;
const int MAXN = 20;
// 棋盘大小
int n;
// 棋盘
char board[MAXN][MAXN];
// 当前玩家
char curPlayer;
// 初始化棋盘
void initBoard() {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
board[i][j] = '-';
}
}
}
// 打印棋盘
void printBoard() {
cout << " ";
for (int i = 0; i < n; i++) {
cout << i + 1 << " ";
}
cout << endl;
for (int i = 0; i < n; i++) {
cout << i + 1 << " ";
for (int j = 0; j < n; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
// 判断当前位置是否为空
bool isEmpty(int x, int y) {
return board[x][y] == '-';
}
// 判断当前位置是否为指定玩家棋子
bool isPlayer(int x, int y, char player) {
return board[x][y] == player;
}
// 落子
void makeMove(int x, int y) {
board[x][y] = curPlayer;
}
// 判断是否有五子连珠
bool hasFiveInRow(int x, int y) {
// 横向
int cnt = 1;
for (int i = y - 1; i >= 0 && isPlayer(x, i, curPlayer); i--) {
cnt++;
}
for (int i = y + 1; i < n && isPlayer(x, i, curPlayer); i++) {
cnt++;
}
if (cnt >= 5) {
return true;
}
// 纵向
cnt = 1;
for (int i = x - 1; i >= 0 && isPlayer(i, y, curPlayer); i--) {
cnt++;
}
for (int i = x + 1; i < n && isPlayer(i, y, curPlayer); i++) {
cnt++;
}
if (cnt >= 5) {
return true;
}
// 斜向(左上到右下)
cnt = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0 && isPlayer(i, j, curPlayer); i--, j--) {
cnt++;
}
for (int i = x + 1, j = y + 1; i < n && j < n && isPlayer(i, j, curPlayer); i++, j++) {
cnt++;
}
if (cnt >= 5) {
return true;
}
// 斜向(左下到右上)
cnt = 1;
for (int i = x + 1, j = y - 1; i < n && j >= 0 && isPlayer(i, j, curPlayer); i++, j--) {
cnt++;
}
for (int i = x - 1, j = y + 1; i >= 0 && j < n && isPlayer(i, j, curPlayer); i--, j++) {
cnt++;
}
if (cnt >= 5) {
return true;
}
return false;
}
// 判断是否有空位置
bool hasEmpty() {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (board[i][j] == '-') {
return true;
}
}
}
return false;
}
// 判断游戏是否结束
bool isGameOver() {
return !hasEmpty() || hasFiveInRow(n - 1, n - 1);
}
// 切换玩家
void switchPlayer() {
curPlayer = curPlayer == 'X' ? 'O' : 'X';
}
int main() {
cout << "请输入棋盘大小:";
cin >> n;
initBoard();
curPlayer = 'X';
while (!isGameOver()) {
printBoard();
int x, y;
do {
cout << "请玩家 " << curPlayer << " 下棋(输入行列数):";
cin >> x >> y;
x--;
y--;
} while (!isEmpty(x, y));
makeMove(x, y);
if (hasFiveInRow(x, y)) {
cout << "玩家 " << curPlayer << " 获胜!" << endl;
break;
}
switchPlayer();
}
printBoard();
cout << "游戏结束!" << endl;
return 0;
}
```
以上代码实现了一个简单的五子棋游戏,包括棋盘初始化、打印棋盘、判断空位置、落子、判断五子连珠、判断游戏是否结束、切换玩家等功能。可以根据需要自行修改和完善。
相关推荐
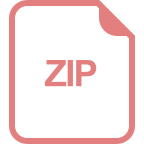
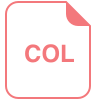
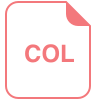












