用C语言编写程序,模拟实现创建新的进程:查看运行进程;换出某个进程:杀死运行进程等功能。
时间: 2024-09-13 20:12:58 浏览: 75
在C语言中,可以使用操作系统提供的系统调用来模拟实现进程的相关操作。以下是一些基本的概念和示例代码片段,用于展示如何在类Unix系统(如Linux)中使用C语言来实现创建新的进程、查看运行进程、换出进程以及杀死运行进程的功能。
1. 创建新的进程:可以使用`fork()`系统调用,它会创建一个新的子进程,该子进程是调用进程的一个副本。在子进程中,`fork()`会返回0,在父进程中,它会返回新创建的子进程的PID。
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork();
if (pid == 0) {
// 子进程代码
printf("子进程的PID是:%d\n", getpid());
} else if (pid > 0) {
// 父进程代码
printf("父进程等待子进程完成\n");
wait(NULL); // 等待子进程结束
} else {
perror("fork失败");
return 1;
}
return 0;
}
```
2. 查看运行进程:可以使用`ps`命令或`/proc`文件系统来获取系统运行进程的信息。在C语言中,可以通过`popen()`函数执行`ps`命令,并读取其输出。
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *fp;
char line[128];
fp = popen("ps -ef", "r");
if (fp == NULL) {
printf("Failed to run command\n" );
exit(1);
}
while (fgets(line, sizeof(line), fp) != NULL) {
printf("%s", line);
}
pclose(fp);
return 0;
}
```
3. 换出某个进程:这通常涉及到进程的调度策略,可以使用`nice`系统调用来改变进程的优先级,使其更可能被换出。不过,具体换出哪个进程还取决于系统的调度器。
```c
#include <stdio.h>
#include <unistd.h>
int main() {
int ret;
ret = nice(1); // 增加当前进程的nice值,降低优先级
if(ret == -1) {
perror("nice failed");
}
return 0;
}
```
4. 杀死运行进程:使用`kill()`系统调用可以向进程发送信号,最常用的信号是`SIGKILL`,它可以强制终止目标进程。
```c
#include <signal.h>
#include <stdio.h>
#include <sys/types.h>
#include <unistd.h>
int main() {
pid_t pid = fork();
if (pid == 0) {
// 子进程代码
} else if (pid > 0) {
// 父进程代码
sleep(5); // 假设运行一段时间后杀死子进程
kill(pid, SIGKILL); // 发送SIGKILL信号杀死子进程
printf("进程 %d 被杀死\n", pid);
} else {
perror("fork失败");
return 1;
}
return 0;
}
```
阅读全文
相关推荐
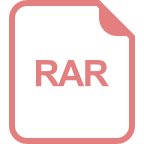
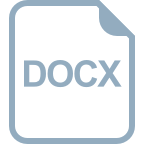
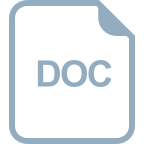














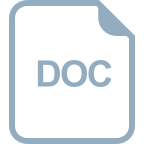
