操作系统用C语言编写程序,模拟实现创建新的进程:查看运行进程;换出某个进程:杀死运行进程等功能。
时间: 2024-09-12 18:09:32 浏览: 176
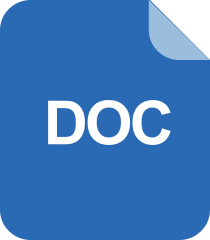
进程创建和杀死
在操作系统中,使用C语言实现进程管理功能是一个复杂但非常基础的任务,这些功能是操作系统核心组件的一部分。下面将简要介绍如何使用C语言来模拟实现创建新进程、查看运行进程、换出某个进程以及杀死运行进程等功能。
1. 创建新的进程(Fork & Exec):
- 在Unix-like系统中,通常使用`fork()`系统调用来创建一个新的进程,该进程是调用进程的一个副本。
- `fork()`后,通常使用`exec()`系列函数来替换当前进程的映像为新的程序执行。
- 这个过程中,父进程可以获取子进程的PID(进程ID),而子进程会获得返回值0,父进程则获得子进程的PID。
- 示例代码片段:
```c
#include <stdio.h>
#include <sys/types.h>
#include <unistd.h>
int main() {
pid_t pid = fork();
if (pid == -1) {
// Fork failed
perror("fork failed");
return 1;
} else if (pid == 0) {
// Child process
printf("This is the child process with PID %d\n", getpid());
// Replace child process with a new program execution
execlp("/bin/ls", "ls", NULL);
} else {
// Parent process
printf("This is the parent process with PID %d and the child PID is %d\n", getpid(), pid);
// Wait for the child process to complete
wait(NULL);
}
return 0;
}
```
2. 查看运行进程:
- 使用`ps`命令可以查看系统中当前运行的进程。
- 在C语言中,可以通过读取`/proc`文件系统或使用`ps`命令的输出来获取进程信息。
- 示例代码片段(读取`/proc`来获取当前进程列表):
```c
#include <stdio.h>
#include <dirent.h>
#include <unistd.h>
int main() {
DIR *dir;
struct dirent *ent;
if ((dir = opendir("/proc")) != NULL) {
while ((ent = readdir(dir)) != NULL) {
// Check if the entry is a directory and a valid PID
if (ent->d_type == DT_DIR) {
char procpath[32];
sprintf(procpath, "/proc/%s", ent->d_name);
DIR *procent = opendir(procpath);
if (procent) {
printf("Process ID: %s\n", ent->d_name);
closedir(procent);
}
}
}
closedir(dir);
} else {
perror("Couldn't open /proc");
}
return 0;
}
```
3. 换出某个进程(Swapping out):
- 操作系统通过页面置换算法将不常用的页面从内存换出到磁盘。
- 在C语言中,一般不会直接实现换出进程的功能,因为这通常是内核级别的操作。
- 但是可以编写代码来释放程序占用的内存资源,例如使用`free()`释放动态分配的内存。
4. 杀死运行进程(Kill):
- 使用`kill`系统调用可以向进程发送信号,最常用的是发送`SIGTERM`信号来请求进程终止。
- 示例代码片段:
```c
#include <signal.h>
#include <sys/types.h>
#include <unistd.h>
int main() {
pid_t pid = ...; // 获取要杀死的进程ID
if (kill(pid, SIGTERM) == -1) {
perror("kill failed");
} else {
printf("Process with PID %d has been signaled to terminate\n", pid);
}
return 0;
}
```
阅读全文
相关推荐
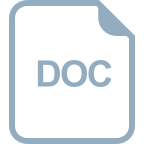
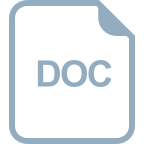






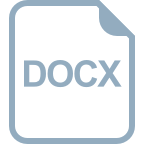
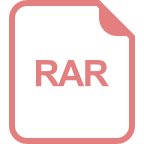
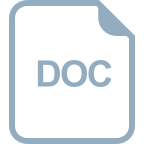
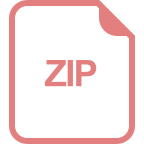
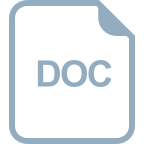
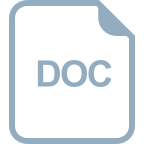
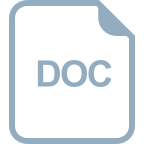
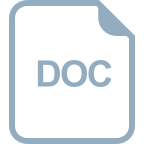
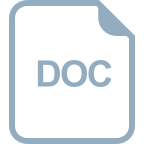
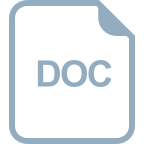