在LINUX系统下用C语言编写一道程序实现进程的管道通信
时间: 2024-05-10 15:16:50 浏览: 9
在 Linux 系统下,可以使用 C 语言中的 `pipe` 函数来创建管道,使用 `fork` 函数来创建子进程,并使用 `dup2` 函数将标准输入和标准输出重定向到管道的读端和写端。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main()
{
int fd[2]; // 管道的文件描述符
pid_t pid;
// 创建管道
if (pipe(fd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
// 创建子进程
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (pid == 0) { // 子进程
close(fd[1]); // 关闭管道的写端
char buf[1024];
int nread;
// 从管道的读端读取数据
while ((nread = read(fd[0], buf, sizeof(buf))) != 0) {
if (nread == -1) {
perror("read");
exit(EXIT_FAILURE);
}
// 将读取到的数据打印到标准输出
if (write(STDOUT_FILENO, buf, nread) == -1) {
perror("write");
exit(EXIT_FAILURE);
}
}
close(fd[0]); // 关闭管道的读端
_exit(EXIT_SUCCESS);
} else { // 父进程
close(fd[0]); // 关闭管道的读端
char *msg = "Hello, world!\n";
// 将数据写入管道的写端
if (write(fd[1], msg, strlen(msg)) == -1) {
perror("write");
exit(EXIT_FAILURE);
}
close(fd[1]); // 关闭管道的写端
// 等待子进程退出
if (wait(NULL) == -1) {
perror("wait");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
}
```
这个程序创建了一个管道,然后创建了一个子进程,子进程从管道的读端读取数据并将其打印到标准输出,父进程将一条消息写入管道的写端,然后等待子进程退出。运行这个程序后,可以看到输出了一条消息 "Hello, world!"。
相关推荐
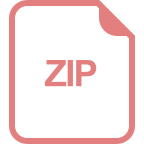
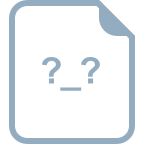














