用c语言编写程序,模拟实现创建新的进程:查看运行进程;换出某个进程;杀死运行进程等功能
时间: 2024-10-15 17:12:41 浏览: 77
在C语言中,要模拟实现创建、查看、挂起(换出)和结束进程的功能,通常会利用系统调用和相关的库函数,如`fork()`, `waitpid()`, `execve()`, 和 `kill()` 等。下面是一个简单的示例,展示了如何实现这些功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
// 创建新进程
int create_new_process(char *command) {
pid_t child_pid = fork();
if (child_pid == 0) { // 子进程
execve(command, command, NULL); // 执行指定命令
perror("Failed to execute");
exit(1);
} else if (child_pid > 0) { // 父进程
printf("Child process created with PID %d\n", child_pid);
} else {
perror("Fork failed");
exit(1);
}
return child_pid;
}
// 查看运行进程
void list_running_processes() {
pid_t status;
while ((status = waitpid(-1, &status, WNOHANG)) > 0) {
printf("Process terminated with PID %d\n", status);
}
if (status == -1 && errno != ECHILD) {
perror("Error waiting for processes");
}
}
// 挂起进程
void suspend_process(pid_t pid) {
if (kill(pid, SIGSTOP) == -1) {
perror("Failed to suspend process");
}
printf("Process %d suspended.\n", pid);
}
// 杀死进程
void kill_process(pid_t pid) {
if (kill(pid, SIGKILL) == -1) {
perror("Failed to kill process");
}
printf("Process %d killed.\n", pid);
}
int main() {
// 示例:创建并启动ls命令的新进程
char* cmd = "/bin/ls";
create_new_process(cmd);
// 查看当前运行进程
list_running_processes();
// 挂起刚刚创建的进程
int child_pid = getppid(); // 获取父进程PID
suspend_process(child_pid);
// 杀死一个进程,这里假设有一个已知的PID
pid_t target_pid = 1234; // 替换成实际需要的PID
kill_process(target_pid);
return 0;
}
```
注意:这个示例仅用于教学目的,实际上在生产环境中,应该避免直接使用`kill()`和`SIGKILL`处理进程管理,而是使用更安全的方式来终止进程,比如通过信号处理程序或者特定的API。
阅读全文
相关推荐
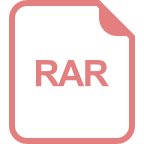
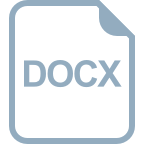
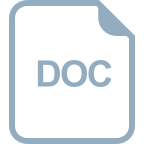








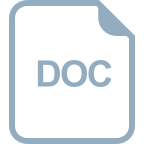

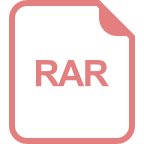
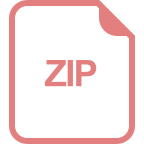
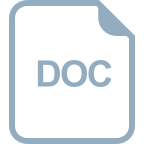
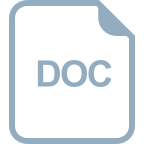
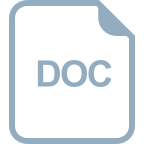
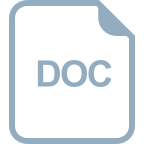