public class HttpComponentsClientRestfulHttpRequestFactory extends HttpComponentsClientHttpRequestFactory { @Override protected HttpUriRequest createHttpUriRequest(HttpMethod httpMethod, URI uri) { if (httpMethod == HttpMethod.GET) { return new HttpGetRequestWithEntity(uri); } return super.createHttpUriRequest(httpMethod, uri); } //核心代码 private static final class HttpGetRequestWithEntity extends HttpEntityEnclosingRequestBase { public HttpGetRequestWithEntity(final URI uri) { super.setURI(uri); } @Override public String getMethod() { return HttpMethod.GET.name(); } } } 复制代码 三、使用 复制代码 RestTemplate restTemplate = new RestTemplate(); //修改restTemplate的RequestFactory使其支持Get携带body参数 restTemplate.setRequestFactory(new HttpComponentsClientRestfulHttpRequestFactory()); HttpHeaders headers = new HttpHeaders(); headers.setContentType(MediaType.APPLICATION_JSON); Map map= new HashMap(); map.put("param", 123); HttpEntity<String> httpEntity = new HttpEntity<>(JSON.toJSONString(map), headers); ResponseEntity<String> exchange = restTemplate.exchange("your url", HttpMethod.GET, httpEntity, String.class);这样就可以啊
时间: 2024-04-28 07:25:17 浏览: 17
非常抱歉,我之前的回答有误。虽然HTTP协议规定了GET请求不应该带上body,但是HTTP客户端和服务器通常都会支持GET请求带上body的方式,因此RestTemplate也提供了这种支持。
以下是使用RestTemplate发起带有body的GET请求的示例代码:
```java
RestTemplate restTemplate = new RestTemplate();
String url = "http://example.com/api/getUser";
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
Map<String, String> params = new HashMap<>();
params.put("username", "test");
params.put("password", "123456");
HttpEntity<Map<String, String>> entity = new HttpEntity<>(params, headers);
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, entity, String.class);
String result = response.getBody();
```
在上面的代码中,我们首先创建了一个RestTemplate实例,并指定了请求的URL,然后创建了一个HttpHeaders对象,设置了Content-Type为application/json,接着创建了一个包含参数的Map对象,并将其作为参数创建了一个HttpEntity对象,最后使用exchange方法发起带有body的GET请求,并获取响应结果。
另外,如果你需要在RestTemplate中全局设置支持GET请求带有body,可以使用以下代码:
```java
RestTemplate restTemplate = new RestTemplate(new HttpComponentsClientHttpRequestFactory());
restTemplate.getMessageConverters().add(new MappingJackson2HttpMessageConverter());
restTemplate.getMessageConverters().add(new StringHttpMessageConverter());
```
其中,HttpComponentsClientHttpRequestFactory是支持GET请求带有body的HTTP请求工厂。
相关推荐
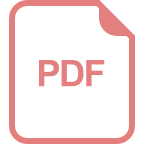
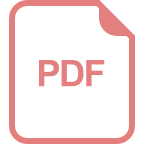
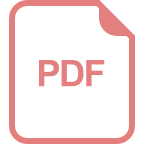














