请用Java实现一个用netty发送http请求的功能
时间: 2024-03-26 11:41:34 浏览: 25
好的,以下是一个使用Netty发送HTTP请求的Java代码示例:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.buffer.Unpooled;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.http.DefaultFullHttpRequest;
import io.netty.handler.codec.http.HttpClientCodec;
import io.netty.handler.codec.http.HttpContent;
import io.netty.handler.codec.http.HttpContentDecompressor;
import io.netty.handler.codec.http.HttpHeaders;
import io.netty.handler.codec.http.HttpMethod;
import io.netty.handler.codec.http.HttpObject;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpRequest;
import io.netty.handler.codec.http.HttpResponse;
import io.netty.handler.codec.http.HttpVersion;
import io.netty.handler.ssl.SslContext;
import io.netty.handler.ssl.SslContextBuilder;
import io.netty.handler.ssl.util.InsecureTrustManagerFactory;
import java.net.URI;
import java.net.URISyntaxException;
public class NettyHttpClient {
private final String host;
private final int port;
private boolean ssl;
private SslContext sslContext;
public NettyHttpClient(String host, int port, boolean ssl) {
this.host = host;
this.port = port;
this.ssl = ssl;
}
public void sendHttpRequest() throws Exception {
NioEventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.TCP_NODELAY, true)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
if (ssl) {
sslContext = SslContextBuilder.forClient()
.trustManager(InsecureTrustManagerFactory.INSTANCE)
.build();
ch.pipeline().addLast(sslContext.newHandler(ch.alloc(), host, port));
}
ch.pipeline().addLast(new HttpClientCodec());
ch.pipeline().addLast(new HttpContentDecompressor());
ch.pipeline().addLast(new HttpObjectAggregator(1024 * 1024));
ch.pipeline().addLast(new HttpClientHandler());
}
});
ChannelFuture f = b.connect(host, port).sync();
URI uri = new URI("http://" + host + ":" + port + "/");
HttpRequest request = new DefaultFullHttpRequest(HttpVersion.HTTP_1_1, HttpMethod.GET, uri.toASCIIString(), Unpooled.EMPTY_BUFFER);
request.headers().set(HttpHeaders.Names.HOST, host);
request.headers().set(HttpHeaders.Names.CONNECTION, HttpHeaders.Values.CLOSE);
request.headers().set(HttpHeaders.Names.ACCEPT_ENCODING, HttpHeaders.Values.GZIP);
f.channel().writeAndFlush(request);
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
private class HttpClientHandler extends SimpleChannelInboundHandler<HttpObject> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, HttpObject msg) throws Exception {
if (msg instanceof HttpResponse) {
HttpResponse response = (HttpResponse) msg;
System.out.println("Status: " + response.getStatus());
System.out.println("Headers: " + response.headers());
}
if (msg instanceof HttpContent) {
HttpContent content = (HttpContent) msg;
System.out.println("Content: " + content.content().toString(io.netty.util.CharsetUtil.UTF_8));
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.close();
}
}
}
```
您可以使用以下代码调用该类:
```java
NettyHttpClient client = new NettyHttpClient("www.example.com", 80, false);
client.sendHttpRequest();
```
该类使用Netty框架实现HTTP请求,包括处理SSL连接。在sendHttpRequest()方法中,我们使用NioEventLoopGroup创建一个新的客户端通道,然后使用Bootstrap配置通道和处理程序。我们创建一个DefaultFullHttpRequest实例来表示HTTP请求,并将其发送到服务器。客户端处理程序接收响应并处理响应中的数据。最后,我们关闭通道并优雅地关闭事件循环组。
相关推荐
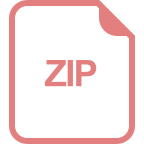
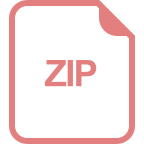
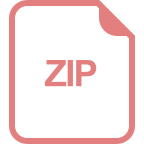














