netty转发http请求
时间: 2023-09-04 13:15:44 浏览: 70
要使用Netty转发HTTP请求,您需要编写一个自定义的ChannelHandler,并将其添加到您的Netty管道中。以下是一个简单的示例:
```java
public class HttpProxyHandler extends ChannelInboundHandlerAdapter {
private final String remoteHost;
private final int remotePort;
public HttpProxyHandler(String remoteHost, int remotePort) {
this.remoteHost = remoteHost;
this.remotePort = remotePort;
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof FullHttpRequest) {
FullHttpRequest request = (FullHttpRequest) msg;
// 创建远程连接
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(ctx.channel().eventLoop())
.channel(NioSocketChannel.class)
.handler(new HttpProxyClientInitializer(ctx.channel()));
ChannelFuture cf = bootstrap.connect(remoteHost, remotePort);
cf.addListener((ChannelFutureListener) future -> {
if (future.isSuccess()) {
// 发送请求
Channel clientChannel = future.channel();
clientChannel.writeAndFlush(request.retain());
// 将代理服务器和客户端之间的连接搭建起来
ctx.pipeline().remove(HttpProxyHandler.this);
clientChannel.pipeline().addLast(new HttpProxyServerHandler(ctx.channel()));
} else {
ctx.channel().close();
}
});
return;
}
ctx.fireChannelRead(msg);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.channel().close();
}
}
```
在上面的例子中,我们创建了一个名为HttpProxyHandler的自定义ChannelHandler。当接收到HTTP请求时,它将建立一个到远程服务器的连接,并将请求转发到该服务器。一旦连接建立成功,它将从管道中删除自己,并添加一个新的ChannelHandler来处理代理服务器和客户端之间的通信。
请注意,此示例仅处理FullHttpRequest,您可能需要根据您的特定用例进行更改。
最后,您需要将此处理程序添加到您的Netty管道中:
```java
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new HttpProxyHandler("localhost", 8080));
}
});
ChannelFuture f = b.bind(8888).sync();
f.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
```
在这个例子中,我们创建了一个简单的代理服务器,它将所有传入的HTTP请求转发到远程服务器(在本例中为localhost:8080)。
希望这个例子能够帮助您开始使用Netty进行HTTP请求转发。
相关推荐
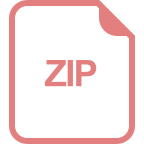
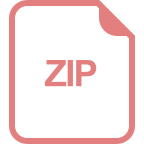













