c# 给某个字段打上正常表达式的标签Attribute
时间: 2024-01-11 13:04:59 浏览: 151
在C#中,可以使用`System.ComponentModel.DataAnnotations`命名空间中的`RegularExpressionAttribute`类来给某个字段打上正则表达式的标签。具体实现可以参考以下代码示例:
```csharp
using System.ComponentModel.DataAnnotations;
public class MyModel
{
[RegularExpression(@"^[a-zA-Z0-9]*$", ErrorMessage = "只能包含字母和数字")]
public string MyField { get; set; }
}
```
在上述代码中,`MyField`字段上使用了`RegularExpressionAttribute`特性,并且指定了正则表达式为`@"^[a-zA-Z0-9]*$"`,错误信息为`"只能包含字母和数字"`。这样,在使用`MyModel`对象时,如果`MyField`字段的值不符合正则表达式,就会提示错误信息。
相关问题
c# 如何通过反射给指定的字段添加Attribute
要给指定的字段添加 Attribute,可以使用 C# 的反射机制来实现。以下是示例代码:
首先,定义一个自定义的 Attribute,如下所示:
```csharp
[AttributeUsage(AttributeTargets.Field)]
public class MyCustomAttribute : Attribute
{
public string Description { get; set; }
public MyCustomAttribute(string description)
{
Description = description;
}
}
```
然后,定义一个包含字段的类:
```csharp
public class MyClass
{
[MyCustom("This is a custom attribute")]
public int MyField;
}
```
接着,使用反射机制获取该类的字段,并给其添加 Attribute:
```csharp
Type myType = typeof(MyClass);
FieldInfo myField = myType.GetField("MyField");
MyCustomAttribute myAttribute = new MyCustomAttribute("This is a custom attribute");
myField.SetCustomAttribute(myAttribute);
```
这样,指定的字段就会被添加上自定义的 Attribute。请注意,Attribute 的使用需要遵循一定的规范,否则可能不会生效。
c#attribute如何限制某些字段必传
我们可以使用自定义的Attribute来限制类的某些字段必须传值,以下是一个示例:
```csharp
[AttributeUsage(AttributeTargets.Property, AllowMultiple = false)]
public class RequiredAttribute : Attribute
{
public string ErrorMessage { get; set; }
public RequiredAttribute(string errorMessage = "This field is required.")
{
ErrorMessage = errorMessage;
}
}
public class User
{
[Required(ErrorMessage = "Name is required.")]
public string Name { get; set; }
[Required(ErrorMessage = "Age is required.")]
public int Age { get; set; }
public string Email { get; set; }
}
public static class Validator
{
public static bool Validate(object obj)
{
var properties = obj.GetType().GetProperties();
foreach (var property in properties)
{
var requiredAttribute = property.GetCustomAttributes(typeof(RequiredAttribute), false).FirstOrDefault() as RequiredAttribute;
if (requiredAttribute != null && property.GetValue(obj) == null)
{
Console.WriteLine(requiredAttribute.ErrorMessage);
return false;
}
}
return true;
}
}
// 使用示例
var user = new User() { Name = "John", Age = 20 };
var isValid = Validator.Validate(user); // isValid = false, 因为Email字段是null
```
在上面的代码中,我们定义了一个自定义的RequiredAttribute,并在User类的Name和Age字段上标记了该Attribute。在Validator类中,我们使用反射获取对象的每个属性,并检查是否存在RequiredAttribute,如果存在并且属性值为null,则返回false。在使用时,我们可以调用Validator.Validate方法来验证对象是否符合要求。
阅读全文
相关推荐
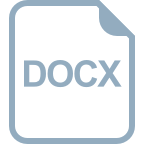
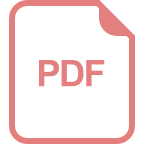
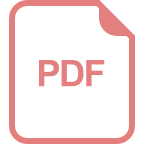













