利用PSO算法解决无碰撞路径模型的python代码
时间: 2023-11-27 15:55:24 浏览: 161
下面是一个基于PSO算法解决无碰撞路径模型的Python代码示例:
```python
import numpy as np
class Particle:
def __init__(self, dim, minx, maxx):
self.__position = np.random.uniform(minx, maxx, dim)
self.__velocity = np.zeros(dim)
self.__best_position = self.__position
self.__best_value = float("inf")
def update_velocity(self, global_best_position, omega, phip, phig):
r1 = np.random.uniform(0, 1, len(self.__position))
r2 = np.random.uniform(0, 1, len(self.__position))
cognitive = phip * r1 * (self.__best_position - self.__position)
social = phig * r2 * (global_best_position - self.__position)
self.__velocity = omega * self.__velocity + cognitive + social
def update_position(self, bounds):
self.__position = np.clip(self.__position + self.__velocity, bounds[:, 0], bounds[:, 1])
def evaluate(self, cost_func):
self.__value = cost_func(self.__position)
if self.__value < self.__best_value:
self.__best_value = self.__value
self.__best_position = self.__position
def get_position(self):
return self.__position
def get_value(self):
return self.__value
class PSO:
def __init__(self, cost_func, dim, population, maxiter, bounds, omega, phip, phig):
self.__cost_func = cost_func
self.__dim = dim
self.__population = [Particle(dim, bounds[:, 0], bounds[:, 1]) for i in range(population)]
self.__maxiter = maxiter
self.__bounds = bounds
self.__omega = omega
self.__phip = phip
self.__phig = phig
self.__best_position = None
self.__best_value = float("inf")
def optimize(self):
for i in range(self.__maxiter):
for particle in self.__population:
particle.evaluate(self.__cost_func)
if particle.get_value() < self.__best_value:
self.__best_value = particle.get_value()
self.__best_position = particle.get_position()
for particle in self.__population:
particle.update_velocity(self.__best_position, self.__omega, self.__phip, self.__phig)
particle.update_position(self.__bounds)
def get_best_position(self):
return self.__best_position
def get_best_value(self):
return self.__best_value
```
其中,`Particle`类表示一个粒子,包含位置、速度和历史最佳位置等属性和方法,`PSO`类表示整个PSO算法,包含粒子群、最大迭代次数、边界等属性和方法。在上述代码中,`cost_func`表示代价函数,`dim`表示参数维度,`population`表示粒子群大小,`maxiter`表示最大迭代次数,`bounds`表示参数边界,`omega`、`phip`和`phig`表示算法的超参数。
在使用时,可以先定义一个代价函数`cost_func`,然后创建一个PSO对象并调用其`optimize`方法进行优化,最后获取最佳位置和最佳值即可。例如,下面是一个用PSO算法求解二维空间中的无碰撞路径模型的示例代码:
```python
def cost_func(x):
# 计算无碰撞路径长度,这里省略具体实现
return length
dim = 2
population = 20
maxiter = 100
bounds = np.array([[-10, 10], [-10, 10]])
omega = 0.5
phip = 0.5
phig = 0.5
pso = PSO(cost_func, dim, population, maxiter, bounds, omega, phip, phig)
pso.optimize()
best_position = pso.get_best_position()
best_value = pso.get_best_value()
print("Best position:", best_position)
print("Best value:", best_value)
```
在上述示例中,我们定义了一个二维的代价函数`cost_func`,然后创建了一个PSO对象并调用了其`optimize`方法进行优化,最后获取到了最佳位置和最佳值。
阅读全文
相关推荐
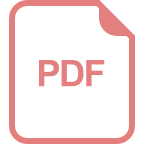
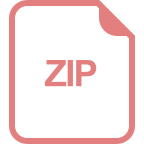



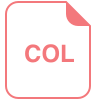
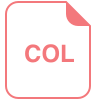
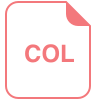
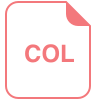
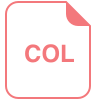
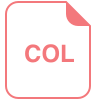
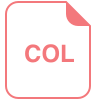
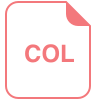
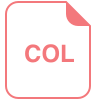
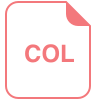
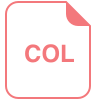
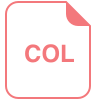
