flask将多个本地图片传到前端展示并下载的前后端代码
时间: 2024-06-10 13:09:22 浏览: 232
很高兴为您解答这个问题!以下是能够实现该功能的 Flask 后端和前端代码:
后端代码:
```python
from flask import Flask, send_file, request, jsonify
import os
app = Flask(__name__)
@app.route('/download', methods=['POST'])
def download():
files = request.files.getlist('files')
for file in files:
file.save(os.path.join('uploads', file.filename))
return jsonify({'message': 'Files uploaded successfully!'})
@app.route('/get_image/<filename>')
def get_image(filename):
return send_file(os.path.join('uploads', filename))
```
这个后端代码会接收前端传来的多个图片文件,保存到本地的 `uploads` 文件夹中,并且提供一个 API `/get_image/<filename>`,可以通过图片文件名获取图片文件。
前端代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Image Uploader and Downloader</title>
</head>
<body>
<form action="/download" method="POST" enctype="multipart/form-data">
<input type="file" name="files" multiple>
<button type="submit">Upload</button>
</form>
<hr>
<div id="images"></div>
<button id="download">Download All Images</button>
<script>
const imagesDiv = document.getElementById('images');
const downloadButton = document.getElementById('download');
fetch('/get_images')
.then(response => response.json())
.then(data => {
data.forEach(filename => {
const img = document.createElement('img');
img.src = `/get_image/${filename}`;
imagesDiv.appendChild(img);
});
});
downloadButton.addEventListener('click', () => {
fetch('/download_all')
.then(response => response.blob())
.then(blob => {
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.href = url;
a.download = 'all_images.zip';
document.body.appendChild(a);
a.click();
a.remove();
});
});
</script>
</body>
</html>
```
这个前端代码会通过上传文件表单将多个图片文件发送给后端,然后使用 `/get_image/<filename>` API 获取每个图片文件的 URL,并且将图片展示在页面上。当用户点击“Download All Images”按钮时,前端会向后端发送 `/download_all` 请求,后端会将所有图片文件打包成一个 ZIP 文件并返回给前端,前端会通过浏览器下载这个 ZIP 文件。
希望这个代码可以帮到您!
阅读全文
相关推荐









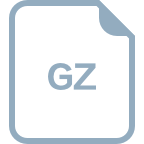






