密码算法的c语言的源代码
时间: 2023-07-19 13:02:10 浏览: 114
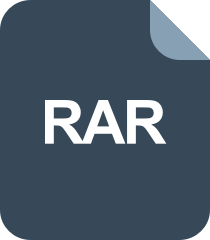
C语言常用算法源代码.rar

### 回答1:
密码算法是一种用于加密和解密敏感数据的算法,常见的密码算法有DES、AES、RSA等。下面是一个使用C语言实现的简单密码算法的源代码示例:
```c
#include <stdio.h>
#include <string.h>
// 简单的密码算法示例
char* simpleEncrypt(char* message, int key) {
int len = strlen(message);
char* encrypted = malloc(len + 1);
for (int i = 0; i < len; i++) {
encrypted[i] = message[i] + key;
}
encrypted[len] = '\0';
return encrypted;
}
char* simpleDecrypt(char* encrypted, int key) {
int len = strlen(encrypted);
char* decrypted = malloc(len + 1);
for (int i = 0; i < len; i++) {
decrypted[i] = encrypted[i] - key;
}
decrypted[len] = '\0';
return decrypted;
}
int main() {
char* message = "Hello, World!";
int key = 3;
// 加密
char* encrypted = simpleEncrypt(message, key);
printf("Encrypted message: %s\n", encrypted);
// 解密
char* decrypted = simpleDecrypt(encrypted, key);
printf("Decrypted message: %s\n", decrypted);
free(encrypted);
free(decrypted);
return 0;
}
```
该示例实现了一个简单的密码算法,使用给定的密钥对消息进行加密和解密。在加密过程中,将每个字符的ASCII码值加上密钥;在解密过程中,将每个字符的ASCII码值减去密钥。这种简单的加密算法只是为了演示目的,实际应用中需要使用更加安全和复杂的密码算法来保护敏感数据。
### 回答2:
密码算法的实现取决于具体的密码算法类型,以下是一个简单的凯撒密码算法的C语言源代码示例:
```c
#include <stdio.h>
#include <string.h>
void caesarCipher(char *text, int key) {
int i;
for (i = 0; i < strlen(text); i++) {
if (text[i] >= 'A' && text[i] <= 'Z') {
// 对大写字母进行位移
text[i] = (text[i] - 'A' + key) % 26 + 'A';
} else if (text[i] >= 'a' && text[i] <= 'z') {
// 对小写字母进行位移
text[i] = (text[i] - 'a' + key) % 26 + 'a';
}
}
}
int main() {
char plaintext[100];
int key;
printf("请输入明文:");
scanf("%s", plaintext);
printf("请输入密钥(位移值):");
scanf("%d", &key);
caesarCipher(plaintext, key);
printf("加密后的密文为:%s\n", plaintext);
return 0;
}
```
这段代码实现了凯撒密码算法,用户输入明文和密钥(位移值),然后调用`caesarCipher`函数对明文进行加密,最后打印加密后的密文。
具体的实现细节是,对输入的明文逐个字符进行遍历,如果是大写字母,则向右位移key个位置,再对26取余;如果是小写字母,则同样进行相同的位移操作。最后将加密后的密文打印出来。
阅读全文
相关推荐
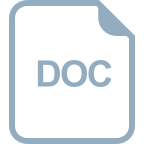
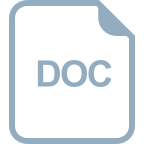
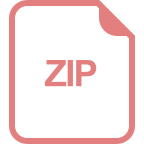
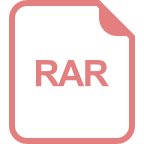
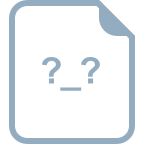
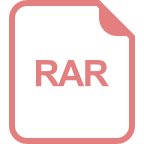
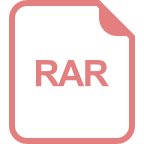
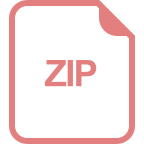
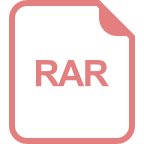
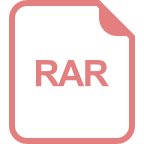
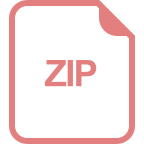
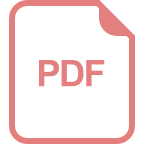
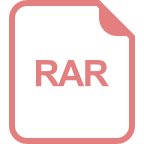