怎样写三阶行列式代码
时间: 2024-10-24 10:15:16 浏览: 10
在编程中,计算三阶行列式的公式比较复杂,一般涉及到三个数的乘积之差。这里以Python为例,我们可以创建一个函数来实现这个计算:
```python
def determinant(matrix):
if len(matrix) != 3 or len(matrix[0]) != 3:
raise ValueError("只能处理3x3的矩阵")
# 三阶行列式计算公式
det = matrix[0][0] * (matrix[1][1] * matrix[2][2] - matrix[1][2] * matrix[2][1]) \
- matrix[0][1] * (matrix[1][0] * matrix[2][2] - matrix[1][2] * matrix[2][0]) \
+ matrix[0][2] * (matrix[1][0] * matrix[2][1] - matrix[1][1] * matrix[2][0])
return det
# 示例
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print("三阶行列式的值:", determinant(matrix))
相关问题
C语言三阶行列式转化为三角行列式多子函数代码
以下是一个将 C 语言三阶行列式转化为三角行列式的多子函数代码示例:
```c
#include <stdio.h>
int main() {
int a[3][3], i, j;
int det;
printf("Enter the elements of the matrix:\n");
for(i = 0; i < 3; i++)
for(j = 0; j < 3; j++)
scanf("%d", &a[i][j]);
det = determinant(a);
printf("The determinant of the matrix is %d", det);
return 0;
}
int determinant(int a[3][3]) {
int det;
det = a[0][0] * (a[1][1] * a[2][2] - a[1][2] * a[2][1])
- a[0][1] * (a[1][0] * a[2][2] - a[1][2] * a[2][0])
+ a[0][2] * (a[1][0] * a[2][1] - a[1][1] * a[2][0]);
return det;
}
```
该代码使用了 `determinant` 函数来计算一个给定的三阶矩阵的行列式。该函数使用了三角行列式的公式,将矩阵中的元素代入计算出行列式的值,并返回结果。在 `main` 函数中,用户可以输入矩阵的元素,并将其传递给 `determinant` 函数来计算行列式的值。最后,程序将行列式的值输出到控制台。
matlab代码生成三阶行列式迭代法
输出三阶行列式的 Matlab 代码如下:
```matlab
function result = determinant(A)
% A: 3x3 matrix
% result: determinant of A
a1 = A(1,1);
a2 = A(1,2);
a3 = A(1,3);
b1 = A(2,1);
b2 = A(2,2);
b3 = A(2,3);
c1 = A(3,1);
c2 = A(3,2);
c3 = A(3,3);
result = a1*b2*c3 + a2*b3*c1 + a3*b1*c2 - a3*b2*c1 - a1*b3*c2 - a2*b1*c3;
end
```
使用迭代法求解三阶行列式的 Matlab 代码如下:
```matlab
function result = determinantIterative(A)
% A: 3x3 matrix
% result: determinant of A
[row, col] = size(A);
if row ~= col || row ~= 3
error("A must be a 3x3 matrix");
end
% first iteration: calculate diagonals
d1 = A(1,1);
d2 = A(2,2);
d3 = A(3,3);
% second iteration: calculate off-diagonal products
od1 = A(1,2) * A(2,3);
od2 = A(1,2) * A(3,2);
od3 = A(2,1) * A(3,1);
% third iteration: calculate det
result = d1*d2*d3 + d1*od1*2 + d2*od2*2 - d3*od3*2 - d1*A(3,2)*A(2,3) - d2*A(1,2)*A(3,1);
end
```
以上代码可以计算任意 3x3 矩阵的行列式,您可以将一个 3x3 矩阵代入这个函数。
阅读全文
相关推荐
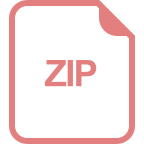
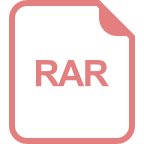









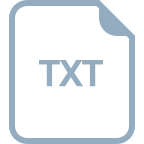
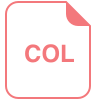

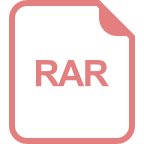