我想用python做一个贪吃蛇游戏
时间: 2023-07-20 21:15:11 浏览: 55
好的,那么你可以使用 Pygame 这个 Python 游戏开发库来实现贪吃蛇游戏。Pygame 提供了丰富的图形、声音、输入等功能,可以方便地实现游戏的各种操作。
以下是一个简单的贪吃蛇游戏的示例代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置游戏窗口大小
width = 640
height = 480
size = (width, height)
screen = pygame.display.set_mode(size)
# 设置窗口标题
pygame.display.set_caption("Snake Game")
# 定义蛇的起始位置和大小
snake_x = width // 2
snake_y = height // 2
snake_size = 20
# 定义蛇的移动速度
snake_speed = 5
# 定义蛇的初始移动方向
snake_direction = "right"
# 定义食物的位置和大小
food_x = random.randint(0, width - snake_size)
food_y = random.randint(0, height - snake_size)
food_size = 20
# 定义分数
score = 0
# 加载字体
font = pygame.font.SysFont(None, 48)
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake_direction = "up"
elif event.key == pygame.K_DOWN:
snake_direction = "down"
elif event.key == pygame.K_LEFT:
snake_direction = "left"
elif event.key == pygame.K_RIGHT:
snake_direction = "right"
# 移动蛇
if snake_direction == "up":
snake_y -= snake_speed
elif snake_direction == "down":
snake_y += snake_speed
elif snake_direction == "left":
snake_x -= snake_speed
elif snake_direction == "right":
snake_x += snake_speed
# 判断是否吃到食物
if snake_x < food_x + food_size and snake_x + snake_size > food_x and \
snake_y < food_y + food_size and snake_y + snake_size > food_y:
food_x = random.randint(0, width - snake_size)
food_y = random.randint(0, height - snake_size)
score += 1
# 绘制游戏界面
screen.fill((255, 255, 255))
pygame.draw.rect(screen, (255, 0, 0), (food_x, food_y, food_size, food_size))
pygame.draw.rect(screen, (0, 255, 0), (snake_x, snake_y, snake_size, snake_size))
# 绘制分数
score_text = font.render("Score: {}".format(score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
# 刷新屏幕
pygame.display.flip()
# 退出 Pygame
pygame.quit()
```
该代码使用 Pygame 实现了一个基本的贪吃蛇游戏,包括蛇的移动、食物的生成、分数的计算和显示等功能。你可以根据自己的需求进行修改和扩展。
相关推荐
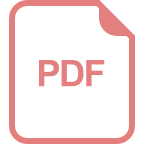
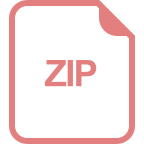













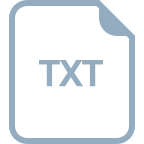