使用JavaFX对汽车风挡玻璃雨刷程序进行可视化模拟操作并写出代码,要求: 1 同一个界面要能够展示两种雨刷的操作(建议使用单选按钮) 2 界面能够仿真驾驶员对控制杆和刻度盘的升/降档操作 3 界面上能够实时显示控制杆档位、刻度盘刻度以及雨刷的摆动速度 4 界面上要能够显示雨刷的摆动动画(仿真),注意,雨刷在形状上要类似于现实中的雨刷器(不是两条线)
时间: 2023-12-06 19:42:12 浏览: 132
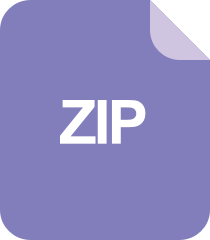
definitive-guide-modern-java-clients-javafx:Stephen Chin,Johan Vos和James Weaver撰写的“使用JavaFX的现代Java客户端权威指南”的源代码-java source code
以下是一个简单的汽车风挡玻璃雨刷程序的JavaFX可视化实现。界面上包含两个单选按钮,用于选择两种不同的雨刷器。控制杆和刻度盘使用滑动条来模拟升/降档操作。界面上实时显示控制杆档位、刻度盘刻度以及雨刷的摆动速度,并能够显示雨刷的摆动动画。
```java
import javafx.animation.KeyFrame;
import javafx.animation.KeyValue;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.RadioButton;
import javafx.scene.control.Slider;
import javafx.scene.control.ToggleGroup;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.util.Duration;
public class WindshieldWiperSimulation extends Application {
private final int WIDTH = 800;
private final int HEIGHT = 600;
private final int MAX_GEAR = 5;
private final int MAX_SPEED = 10;
private ImageView wiper1;
private ImageView wiper2;
private Label gearLabel;
private Label speedLabel;
private int currentGear = 0;
private int currentSpeed = 0;
@Override
public void start(Stage primaryStage) {
BorderPane root = new BorderPane();
Scene scene = new Scene(root, WIDTH, HEIGHT);
// Create image views for windshield wipers
Image wiperImage = new Image("wiper.png");
wiper1 = new ImageView(wiperImage);
wiper2 = new ImageView(wiperImage);
wiper1.setFitWidth(300);
wiper1.setPreserveRatio(true);
wiper2.setFitWidth(300);
wiper2.setPreserveRatio(true);
// Create radio buttons for selecting wiper type
RadioButton wiper1Button = new RadioButton("Wiper 1");
RadioButton wiper2Button = new RadioButton("Wiper 2");
ToggleGroup wiperGroup = new ToggleGroup();
wiper1Button.setToggleGroup(wiperGroup);
wiper2Button.setToggleGroup(wiperGroup);
wiper1Button.setSelected(true);
// Create sliders for gear and speed control
Slider gearSlider = new Slider(0, MAX_GEAR, 0);
gearSlider.setMajorTickUnit(1);
gearSlider.setSnapToTicks(true);
Slider speedSlider = new Slider(0, MAX_SPEED, 0);
speedSlider.setMajorTickUnit(1);
speedSlider.setSnapToTicks(true);
// Add labels for gear and speed
gearLabel = new Label("Gear: 0");
speedLabel = new Label("Speed: 0");
// Add sliders and labels to hbox
HBox controlBox = new HBox(20,
new VBox(10, new Label("Gear"), gearSlider, gearLabel),
new VBox(10, new Label("Speed"), speedSlider, speedLabel));
controlBox.setAlignment(Pos.CENTER);
controlBox.setPadding(new Insets(20));
// Add radio buttons and control hbox to vbox
VBox radioBox = new VBox(20, wiper1Button, wiper2Button, controlBox);
radioBox.setAlignment(Pos.CENTER);
radioBox.setPadding(new Insets(20));
// Add radio vbox and wiper image views to borderpane
root.setLeft(radioBox);
HBox wiperBox = new HBox(wiper1, wiper2);
wiperBox.setAlignment(Pos.CENTER);
root.setCenter(wiperBox);
// Add listeners to gear and speed sliders
gearSlider.valueProperty().addListener((obs, oldVal, newVal) -> {
currentGear = newVal.intValue();
gearLabel.setText("Gear: " + currentGear);
updateSpeed();
updateWiperSpeed();
});
speedSlider.valueProperty().addListener((obs, oldVal, newVal) -> {
currentSpeed = newVal.intValue();
speedLabel.setText("Speed: " + currentSpeed);
updateWiperSpeed();
});
primaryStage.setTitle("Windshield Wiper Simulation");
primaryStage.setScene(scene);
primaryStage.show();
}
/**
* Update the calculated speed based on gear and speed settings.
*/
private void updateSpeed() {
currentSpeed = currentGear * 2;
if (currentSpeed > MAX_SPEED) {
currentSpeed = MAX_SPEED;
}
}
/**
* Update the wiper speed based on current speed setting.
*/
private void updateWiperSpeed() {
double angle = currentSpeed * 15;
// Set animation timeline for wiper 1
Timeline timeline1 = new Timeline(
new KeyFrame(Duration.ZERO, new KeyValue(wiper1.rotateProperty(), 0)),
new KeyFrame(Duration.seconds(0.5), new KeyValue(wiper1.rotateProperty(), angle))
);
timeline1.play();
// Set animation timeline for wiper 2
Timeline timeline2 = new Timeline(
new KeyFrame(Duration.ZERO, new KeyValue(wiper2.rotateProperty(), 0)),
new KeyFrame(Duration.seconds(0.5), new KeyValue(wiper2.rotateProperty(), -angle))
);
timeline2.play();
}
}
```
在代码中,我们使用了JavaFX的各种布局和控件,以及动画效果来实现汽车风挡玻璃雨刷程序的可视化。可以根据需要进行调整和扩展。
阅读全文
相关推荐
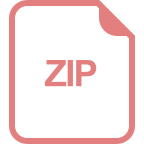
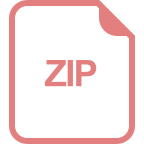


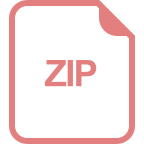
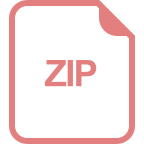
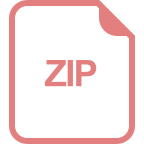
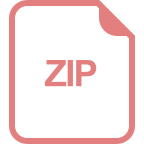
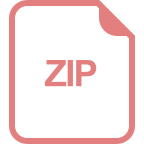
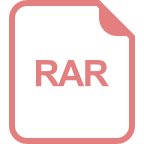
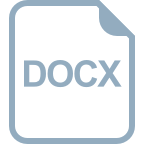
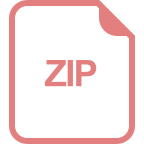
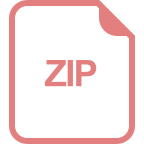
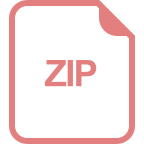
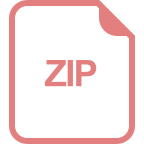
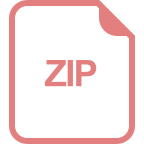
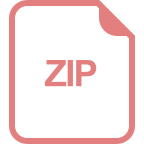
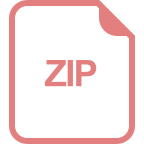