用从c++写出代码:给定一个字符串,找到它的第一个不重复的字符,并返回它的索引。如果不存在,则返回 -1。
时间: 2023-12-03 11:46:14 浏览: 31
以下是用 c++ 实现的解决方案:
```c++
#include <iostream>
#include <unordered_map>
using namespace std;
int firstUniqChar(string s) {
unordered_map<char, int> charMap;
for (char c : s) {
charMap[c]++;
}
for (int i = 0; i < s.length(); i++) {
if (charMap[s[i]] == 1) {
return i;
}
}
return -1;
}
int main() {
string s = "leetcode";
int index = firstUniqChar(s);
cout << index << endl; // 输出 0
return 0;
}
```
此代码中,我们使用了一个哈希表来记录每个字符出现的次数。然后我们遍历一遍字符串,找到第一个出现次数为 1 的字符,返回其索引即可。如果遍历完整个字符串没有找到这样的字符,就返回 -1。
相关问题
写一个c++代码 给定一个字符串,找到它的第一个不重复的字符,并返回它的索引。如果不存在,则返回 -1。
下面是C++的实现代码:
```c++
#include <iostream>
#include <unordered_map>
using namespace std;
int firstUniqChar(string s) {
unordered_map<char, int> freq;
for (char c : s) {
freq[c]++;
}
for (int i = 0; i < s.size(); i++) {
if (freq[s[i]] == 1) {
return i;
}
}
return -1;
}
int main() {
string s = "leetcode";
int res = firstUniqChar(s);
cout << res << endl; // 输出 0
return 0;
}
```
该算法的时间复杂度是O(n),其中n是字符串的长度。首先,我们使用一个哈希表记录每个字符在字符串中出现的次数。然后,我们遍历字符串,找到第一个出现次数为1的字符,返回其索引。如果没有找到这样的字符,则返回-1。
给定一个字符串,找到它的第一个不重复的字符,并返回它的索引。如果不存在,则返回 -1。用DEVc++实现
好的,下面是 DEV-C++ 的代码实现:
```cpp
#include <iostream>
#include <string>
#include <unordered_map>
using namespace std;
int firstUniqChar(string s) {
unordered_map<char, int> charCount;
for (char c : s) {
charCount[c]++;
}
for (int i = 0; i < s.length(); i++) {
if (charCount[s[i]] == 1) {
return i;
}
}
return -1;
}
int main() {
string s = "leetcode";
int result = firstUniqChar(s);
cout << result << endl; // 输出 0
return 0;
}
```
这个函数的实现和上面的 C++ 代码是一样的。你可以在 DEV-C++ 中创建一个新的 C++ 项目,然后将这个代码复制到 `main.cpp` 中,然后编译运行即可。
相关推荐
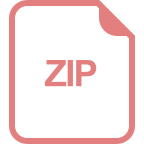
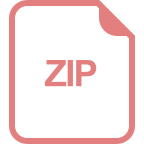
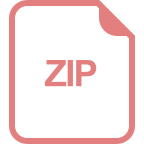
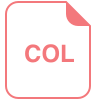
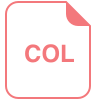
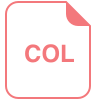
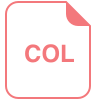
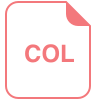







