java贪吃蛇代码eclipse
时间: 2023-07-02 21:10:11 浏览: 65
以下是使用Eclipse编写的Java贪吃蛇代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Snake extends JFrame implements ActionListener {
private static final long serialVersionUID = 6678292058307426314L;
private JLabel label;
private JButton startButton, exitButton;
private Timer timer;
private JPanel panel;
private int x[] = new int[400], y[] = new int[400];
private int len = 3, foodx, foody, width = 10, height = 10, direction = 1;
private boolean dead = false, pause = false;
public Snake() {
super("贪吃蛇");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 400);
setLocationRelativeTo(null);
setResizable(false);
panel = new JPanel();
panel.setLayout(null);
label = new JLabel("分数: 0");
label.setBounds(5, 5, 100, 20);
panel.add(label);
startButton = new JButton("开始");
startButton.setBounds(120, 5, 80, 20);
startButton.addActionListener(this);
panel.add(startButton);
exitButton = new JButton("退出");
exitButton.setBounds(220, 5, 80, 20);
exitButton.addActionListener(this);
panel.add(exitButton);
add(panel);
setVisible(true);
Graphics g = panel.getGraphics();
g.setColor(Color.WHITE);
g.fillRect(0, 0, 400, 400);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
startButton.setEnabled(false);
timer = new Timer(100, this);
timer.start();
init();
draw();
addfood();
return;
}
if (e.getSource() == exitButton) {
System.exit(0);
}
if (e.getSource() == timer) {
if (!dead) {
move();
draw();
} else {
timer.stop();
int result = JOptionPane.showConfirmDialog(this, "游戏结束!是否重新开始?", "游戏结束", JOptionPane.YES_NO_OPTION);
if (result == JOptionPane.YES_OPTION) {
init();
draw();
addfood();
timer.start();
dead = false;
} else {
System.exit(0);
}
}
}
}
public void init() {
for (int i = 0; i < len; i++) {
x[i] = (len - i - 1) * width + 50;
y[i] = 50;
}
len = 3;
direction = 1;
dead = false;
pause = false;
label.setText("分数: 0");
}
public void draw() {
Graphics g = panel.getGraphics();
g.setColor(Color.WHITE);
g.fillRect(0, 0, 400, 400);
g.setColor(Color.RED);
g.fillRect(foodx, foody, width, height);
for (int i = 0; i < len; i++) {
g.setColor(Color.GREEN);
g.fillRect(x[i], y[i], width, height);
}
}
public void addfood() {
int fx = (int) (Math.random() * 38) * 10 + 10;
int fy = (int) (Math.random() * 38) * 10 + 10;
foodx = fx;
foody = fy;
}
public void move() {
for (int i = len; i > 0; i--) {
x[i] = x[i - 1];
y[i] = y[i - 1];
}
if (direction == 0) {
y[0] -= height;
}
if (direction == 1) {
x[0] += width;
}
if (direction == 2) {
y[0] += height;
}
if (direction == 3) {
x[0] -= width;
}
if (x[0] == foodx && y[0] == foody) {
len++;
label.setText("分数: " + (len - 3) * 10);
addfood();
}
if (x[0] < 0 || x[0] > 390 || y[0] < 0 || y[0] > 390) {
dead = true;
}
for (int i = 1; i < len; i++) {
if (x[0] == x[i] && y[0] == y[i]) {
dead = true;
}
}
}
public static void main(String args[]) {
new Snake();
}
}
```
运行该程序,在窗口中点击开始按钮即可开始游戏。使用方向键控制蛇的移动方向,吃到食物后蛇的长度会增加,游戏结束后可以选择重新开始或退出游戏。
相关推荐
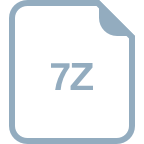












