vue二次封装一些工具方法
时间: 2023-10-29 18:00:03 浏览: 153
好的,我可以帮你封装一些常用的工具方法。以下是一个示例,你可以根据自己的需求进行修改和扩展:
```javascript
// utils.js
// 格式化日期
export function formatDate(date) {
const options = { year: 'numeric', month: 'long', day: 'numeric' };
return new Date(date).toLocaleDateString(undefined, options);
}
// 防抖函数
export function debounce(func, delay) {
let timer;
return function(...args) {
clearTimeout(timer);
timer = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
// 深拷贝对象
export function deepClone(obj) {
if (obj === null || typeof obj !== 'object') {
return obj;
}
let clone = Array.isArray(obj) ? [] : {};
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
clone[key] = deepClone(obj[key]);
}
}
return clone;
}
// 获取随机整数
export function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1)) + min;
}
```
你可以在 Vue 组件或其他地方导入这些工具方法并使用它们:
```javascript
import { formatDate, debounce, deepClone, getRandomInt } from './utils';
export default {
data() {
return {
inputValue: '',
};
},
methods: {
handleInput: debounce(function() {
// 处理输入事件,使用防抖函数
}, 300),
handleClick() {
const clonedData = deepClone(this.data);
const randomInt = getRandomInt(1, 10);
const formattedDate = formatDate(new Date());
// 其他操作
},
},
};
```
这只是一个简单的例子,你可以根据实际需要进行修改和扩展。希望能对你有所帮助!如果有任何问题,请随时问我。
阅读全文
相关推荐
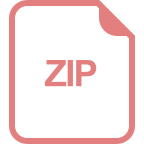
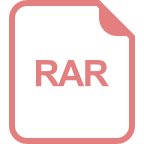
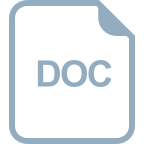
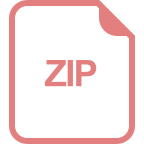
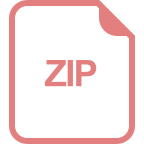
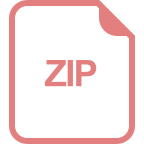
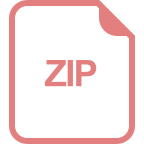
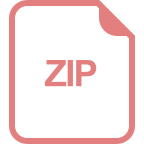
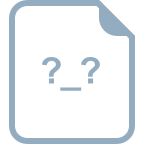
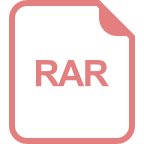
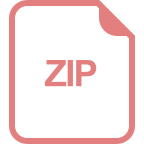
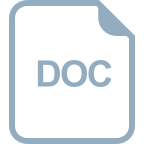
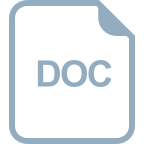



