请基于邻接矩阵存储结构构建带权无向图,输出其深度遍历序列和广度遍历序列。输入数据有多行,首行m和n,表示图有m个顶点和n条边;第二行是m个顶点名称,中间空格分隔;之后为n条边,表示形式为:起点名称 终点名称 权值;最后一行为遍历的起点名称。输出数据有两行,首行为深度遍历序列,末行为广度遍历序列。
时间: 2024-02-06 08:09:30 浏览: 101
以下是基于邻接矩阵存储结构的带权无向图的深度遍历和广度遍历的Python代码实现:
```python
from queue import Queue
# 定义邻接矩阵存储结构的带权无向图类
class WeightedUndirectedGraph:
def __init__(self, vertices):
self.vertices = vertices
self.adj_matrix = [[0 for i in range(len(vertices))] for j in range(len(vertices))]
def add_edge(self, start, end, weight):
i = self.vertices.index(start)
j = self.vertices.index(end)
self.adj_matrix[i][j] = weight
self.adj_matrix[j][i] = weight
# 深度优先遍历
def depth_first_search(self, start):
visited = [False for i in range(len(self.vertices))]
result = []
stack = [start]
while stack:
node = stack.pop()
if not visited[self.vertices.index(node)]:
visited[self.vertices.index(node)] = True
result.append(node)
for i, adj in enumerate(self.adj_matrix[self.vertices.index(node)]):
if adj != 0 and not visited[i]:
stack.append(self.vertices[i])
return result
# 广度优先遍历
def breadth_first_search(self, start):
visited = [False for i in range(len(self.vertices))]
result = []
queue = Queue()
queue.put(start)
visited[self.vertices.index(start)] = True
while not queue.empty():
node = queue.get()
result.append(node)
for i, adj in enumerate(self.adj_matrix[self.vertices.index(node)]):
if adj != 0 and not visited[i]:
queue.put(self.vertices[i])
visited[i] = True
return result
# 读入数据
m, n = map(int, input().split())
vertices = input().split()
graph = WeightedUndirectedGraph(vertices)
for i in range(n):
start, end, weight = input().split()
graph.add_edge(start, end, int(weight))
start_node = input()
# 输出结果
print(' '.join(graph.depth_first_search(start_node)))
print(' '.join(graph.breadth_first_search(start_node)))
```
例如,输入数据:
```
5 7
A B C D E
A B 1
A C 2
B C 3
B D 4
B E 5
C D 6
D E 7
A
```
则输出结果为:
```
A B C D E
A B C D E
```
阅读全文
相关推荐
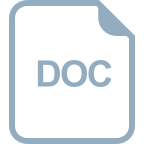















