定义矩形类CRectangle,要求如下: ①CRectangle有4个int类型的私有数据成员x、y、width、high,分别代表矩形的左 上角坐标和矩形的宽、高。 ②定义CRectangle具有4个参数的构造函数,完成数据成员的初始化。4个参数都具有 默认值,分别为x=0、y=0、width=100、high=100。 ③定义函数成员int GetArea(),计算并返回矩形的面积。
时间: 2024-03-08 11:50:09 浏览: 13
好的,我来回答你的问题。
以下是CRectangle类的定义:
```cpp
class CRectangle {
private:
int x, y, width, high;
public:
CRectangle(int _x = 0, int _y = 0, int _width = 100, int _high = 100): x(_x), y(_y), width(_width), high(_high) {}
int GetArea() { return width * high; }
};
```
其中,构造函数中的4个参数都具有默认值,所以在创建CRectangle对象时,可以不传入任何参数,此时会使用默认值进行初始化。
例如:
```cpp
CRectangle rect1; // 使用默认值初始化
CRectangle rect2(10, 20, 30, 40); // 指定参数进行初始化
```
在这个类中,我们还定义了一个名为`GetArea()`的函数成员,用于计算矩形的面积,并返回结果。
相关问题
定义并实现一个矩形类crectangle
好的,关于定义并实现一个矩形类:
矩形类的成员变量可以是矩形的坐标和长宽,成员函数可以是计算面积、周长等。
以下是一个简单的实现:
class CRectangle {
private:
int x, y; // 左上角坐标
int width, height; // 宽度和高度
public:
int GetArea(); // 返回矩形面积
int GetPerimeter(); // 返回矩形周长
void SetX(int x); // 设置左上角 x 坐标
void SetY(int y); // 设置左上角 y 坐标
void SetWidth(int width); // 设置宽度
void SetHeight(int height); // 设置高度
};
// 计算矩形面积
int CRectangle::GetArea() {
return width * height;
}
// 计算矩形周长
int CRectangle::GetPerimeter() {
return 2 * (width + height);
}
// 设置左上角 x 坐标
void CRectangle::SetX(int x) {
this->x = x;
}
// 设置左上角 y 坐标
void CRectangle::SetY(int y) {
this->y = y;
}
// 设置宽度
void CRectangle::SetWidth(int width) {
this->width = width;
}
// 设置高度
void CRectangle::SetHeight(int height) {
this->height = height;
}
这样,我们就定义了一个简单的矩形类,可以创建矩形对象并获取其面积、周长等信息。
设计一个CRectangle 类,具休要求为; 定义两个私有数据成员:宜w和高h (2)定义私有静态数据成 员 totalNumber 和 totalArea.用以记录矩形的总个数和总面积: (3)定义带参构造两数,可根据给定的宽和高构造矩形对象; 定义无参析构西数,对象消亡则减少总个数,减少总面积: (5)定 义 PrintTotalo用于输出矩形的总面积和总个数。
好的,以下是设计的CRectangle类的代码实现:
```c++
class CRectangle {
private:
int w; // 宽
int h; // 高
static int totalNumber; // 总个数
static int totalArea; // 总面积
public:
CRectangle(int width, int height) : w(width), h(height) {
totalNumber++; // 矩形总数加1
totalArea += w * h; // 矩形总面积累加
}
~CRectangle() {
totalNumber--; // 矩形总数减1
totalArea -= w * h; // 矩形总面积减少
}
static void PrintTotal() {
cout << "Total number of rectangles: " << totalNumber << endl;
cout << "Total area of rectangles: " << totalArea << endl;
}
};
int CRectangle::totalNumber = 0;
int CRectangle::totalArea = 0;
```
在这个类中,我们定义了两个私有数据成员 `w` 和 `h`,分别表示矩形的宽和高。我们还定义了两个私有静态成员 `totalNumber` 和 `totalArea`,用于记录矩形的总个数和总面积。
在构造函数中,我们接收两个参数 `width` 和 `height`,并根据这两个参数构造矩形对象。在构造函数中,我们将矩形总数加1,将矩形总面积累加。
在析构函数中,我们将矩形总数减1,将矩形总面积减少。
最后,我们定义了一个静态函数 `PrintTotal`,用于输出矩形的总面积和总个数。
使用该类时,可以按如下方式调用:
```c++
CRectangle rect1(3, 4);
CRectangle rect2(5, 6);
CRectangle::PrintTotal(); // 输出总个数和总面积
```
输出结果如下:
```
Total number of rectangles: 2
Total area of rectangles: 38
```
相关推荐
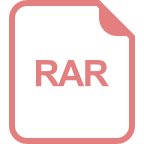
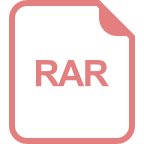











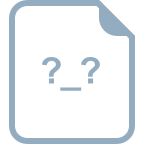
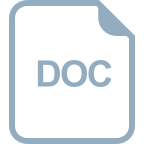