with open('maoyan100-02.csv',mode='a',newline="",encoding='utf-8') as f: csv_writer = csv.writer(f) csv_writer.writerow([title,star,releasetime,score])
时间: 2023-12-08 21:06:16 浏览: 28
这段代码是使用Python内置的csv模块来写入一个CSV文件。首先使用`open()`函数打开一个文件,指定文件名为`maoyan100-02.csv`,使用`mode='a'`来表示以追加方式打开文件(即如果文件存在,就在文件末尾追加内容;如果文件不存在,就创建一个新文件并写入内容)。接着使用`csv.writer()`函数创建一个csv写入器,将文件对象`f`传入其中。然后使用`csv_writer.writerow()`方法来写入一行数据,这里写入了4个字段:`title`、`star`、`releasetime`和`score`。注意,这里写入的每个字段都需要用列表或元组的方式传入。最后使用`with`语句来自动关闭文件。
相关问题
import requests import pprint url = 'http://piaofang.maoyan.com/dashboard-ajax/movie' params = {} cookies = {} headers = {} response = requests.get(url=url, params=params, headers=headers, cookies=cookies) html_data = response.json() pprint.pprint(html_data) movieList = html_data['movieList']['list'] dit = {} for i in movieList: dit['电影名'] = i['movieInfo']['movieName'] dit['票房'] = i['sumBoxDesc'] dit['票房占比'] = i['boxRate'] dit['排片占比'] = i['showCountRate'] dit['上映周期'] = i['movieInfo']['releaseInfo'] pprint.pprint(dit) import csv f = open('data.csv', mode='a', encoding='utf-8', newline='') csv_write = csv.DictWriter(f, fieldnames=['电影名', '票房', '票房占比', '排片占比', '上映周期']) csv_write.writeheader() f.close()
这段代码是用Python爬取猫眼电影的实时票房数据,并将数据写入到CSV文件中。首先使用requests库向猫眼电影的接口发送请求,获取到返回的JSON数据,然后使用pprint库将JSON数据格式化输出。接着,从JSON数据中提取电影列表,并循环遍历电影列表,将电影名、票房、票房占比、排片占比、上映周期等信息存储到字典中。然后使用csv库将这些字典数据写入到CSV文件中。最后,关闭文件。
import requests from bs4 import BeautifulSoup import openpyxl def get_movie_data(year): url = f'https://maoyan.com/films?year={year}' headers = {'User-Agent': 'Mozilla/5.0'} response = requests.get(url, headers=headers) if response.status_code == 200: soup = BeautifulSoup(response.content, 'html.parser') movies = soup.select('.movie-item-title') movie_data = [] for movie in movies: movie_link = 'https://maoyan.com' + movie.a['href'] movie_data.append(get_movie_details(movie_link)) return movie_data else: print(f"Failed to fetch data for year {year}") return [] def get_movie_details(url): headers = {'User-Agent': 'Mozilla/5.0'} response = requests.get(url, headers=headers) if response.status_code == 200: soup = BeautifulSoup(response.content, 'html.parser') movie_name = soup.select_one('h1.name').text.strip() release_date = soup.select_one('.info-release').text.strip() genre = soup.select_one('.info-category').text.strip() director = soup.select_one('.info-director').text.strip() actors = [actor.text.strip() for actor in soup.select('.info-actor a')] maoyan_score = soup.select_one('.score-num').text.strip() box_office = soup.select_one('.info-num').text.strip() return { '电影名称': movie_name, '上映日期': release_date, '影片类型': genre, '导演': director, '演员': ', '.join(actors), '猫眼口碑': maoyan_score, '累计票房': box_office } else: print(f"Failed to fetch details for {url}") return {} def save_to_excel(data, filename): wb = openpyxl.Workbook() ws = wb.active headers = ['电影名称', '上映日期', '影片类型', '导演', '演员', '猫眼口碑', '累计票房'] ws.append(headers) for movie in data: row_data = [movie.get(header, '') for header in headers] ws.append(row_data) wb.save(filename) print(f"Data saved to {filename}") if __name__ == '__main__': years = range(2017, 2021) all_movie_data = [] for year in years: movie_data = get_movie_data(year) all_movie_data.extend(movie_data) save_to_excel(all_movie_data, 'maoyan_movies_2017_to_2020.xlsx')
这段代码是一个Python脚本,用于从猫眼电影网站上爬取指定年份的电影数据,并保存到Excel文件中。
代码使用了requests库来发送HTTP请求,使用BeautifulSoup库来解析HTML内容,使用openpyxl库来操作Excel文件。
主要功能包括:
- `get_movie_data(year)`函数:根据指定年份获取电影数据的函数。
- `get_movie_details(url)`函数:获取电影详情的函数。
- `save_to_excel(data, filename)`函数:将电影数据保存到Excel文件的函数。
在主程序中,通过循环遍历2017年到2020年的年份,调用`get_movie_data(year)`函数获取电影数据,然后将所有电影数据保存到名为`maoyan_movies_2017_to_2020.xlsx`的Excel文件中。
注意:爬取网站数据时,请遵守网站的相关规定和法律法规,不要过度请求或滥用。
相关推荐
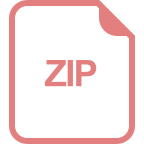
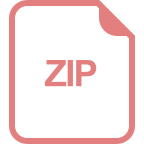




用正则表达式.?board-index.?>(.?).?data-src="(.?)".?name.?a.?>(.?)
.?star.?>(.?).?releasetime.?>(.?).?integer.?>(.?).?fraction.?>(.?).?爬取猫眼电影排行:爬取猫眼电影TOP100的所有信息。网址:https://www.maoyan.com/board/4 
用正则表达式.?board-index.?>(.?).?data-src="(.?)".?name.?a.?>(.?)
.?star.?>(.?).?releasetime.?>(.?).?integer.?>(.?).?fraction.?>(.?).?爬取猫眼电影TOP100的所有信息。网址:https://www.maoyan.com/board/4 







