using namespace std; const int maxn = 205; const int INF = 0x3f3f3f3f; int d[maxn][maxn]; int terminal[maxn], vis[maxn][maxn]; map<int, int> been[maxn]; int n, m, k; int line[10000];
时间: 2023-07-31 22:06:34 浏览: 106
下面是将该段代码转换为C语言的过程:
```c
#include <stdio.h>
#include <map.h>
const int maxn = 205;
const int INF = 0x3f3f3f3f;
int d[maxn][maxn];
int terminal[maxn], vis[maxn][maxn];
map<int, int> been[maxn];
int n, m, k;
int line[10000];
int main() {
// code here ...
return 0;
}
```
需要注意的细节有:
- C语言中没有 `using namespace std;` 这样的语句,需要手动使用 `std::` 前缀来访问 `namespace` 中的成员。
- `map` 属于 C++ STL 中的容器,C语言中没有类似的容器。可以使用 `unordered_map` 来代替 `map`,或者手动实现一个哈希表。
- `const` 关键字也需要在 C语言中使用。
- 变量的定义和类型需要根据题目而定,例如 `line` 数组的大小和类型需要根据题目而定。
相关问题
#include <iostream>#include <ctime>#include <cstdlib>using namespace std;const int MAXN = 500; // 需要排序的数组大小const int MAXV = 2000; // 随机数的最大值// 直接插入排序void insertSort(int a[], int
Sure, I can help you with that. The code you provided is written in C++ and it seems to be implementing the insertion sort algorithm. Insertion sort is a simple sorting algorithm that works by repeatedly inserting elements into a sorted portion of the array.
Here's the complete implementation of the insertion sort algorithm based on the code you provided:
```cpp
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
const int MAXN = 500; // Size of the array to be sorted
const int MAXV = 2000; // Maximum value for random numbers
// Function to generate random numbers
void generateRandomArray(int a[], int n) {
srand(time(0));
for (int i = 0; i < n; i++) {
a[i] = rand() % MAXV;
}
}
// Function to print the array
void printArray(int a[], int n) {
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
}
// Insertion sort implementation
void insertionSort(int a[], int n) {
for (int i = 1; i < n; i++) {
int key = a[i];
int j = i - 1;
while (j >= 0 && a[j] > key) {
a[j + 1] = a[j];
j--;
}
a[j + 1] = key;
}
}
int main() {
int arr[MAXN];
// Generate random array
generateRandomArray(arr, MAXN);
cout << "Original array: ";
printArray(arr, MAXN);
// Sort the array using insertion sort
insertionSort(arr, MAXN);
cout << "Sorted array: ";
printArray(arr, MAXN);
return 0;
}
```
This code generates a random array of size `MAXN` with values ranging from 0 to `MAXV`. It then sorts the array using the insertion sort algorithm and prints the original and sorted arrays.
Let me know if you have any further questions or if there's anything else I can assist you with!
const int maxn=100010作用
### 回答1:
const int maxn=100010的作用是定义一个常量maxn,其值为100010。在程序中,可以使用这个常量来代替具体的数值,使代码更加清晰易懂,同时也方便修改和维护。例如,在数组定义中,可以使用maxn来表示数组的最大长度,而不需要在多个地方重复写出100010这个数值。
### 回答2:
const int maxn=100010是一个常量的定义,它的作用是为了规定一个数组的最大长度,方便在编写程序时使用。
在计算机程序设计中,常量是指在整个程序运行过程中,其值不变的变量。当我们需要使用常量时,常常采用宏或变量的方式进行定义。但是,在宏定义的情况下,我们无法确保常量的类型和值,而且不同的宏定义会造成代码的不可读性和维护困难。在变量定义的情况下,我们可以通过变量赋值或修改来改变常量的值,影响程序的正确性和可维护性。
因此,const常量的定义方式是更加严格和安全的方法。“const”关键字的作用是定义一个具有特定类型和初始值的常量,并且在程序运行时,其值不能修改。const常量的定义方式具有以下特点:
1. 类型安全性:const常量的类型在定义时就已经确定了,避免了在宏定义时类型错误的问题。
2. 可读性:const常量的定义方式代码结构更加清晰,常量的含义和使用也更加容易理解。
在const int maxn=100010中,maxn是一个常量,类型为int,初始值为100010。这个常量通常用在对数组进行定义时,规定数组的最大长度,避免越界和内存泄漏的问题。使用const int maxn=100010的好处是,即使在程序的其他部分更改maxn的值也不会影响到程序中前面使用该常量的其他部分,从而保证了程序的正确性和稳定性。
总之,const int maxn=100010的作用是定义一个类型安全、可读性强的常量,用于规定数组的最大长度,从而确保程序的正确性和稳定性。
### 回答3:
程序设计语言中,常数(constant)是指在程序运行过程中,值保持不变的标识符。C++语言支持常数的定义,定义方法是使用关键字const。在C++中,const常量也可以定义为数组大小,比如const int maxn=100010。
const int maxn=100010的作用是定义了一个常数maxn,且值为100010。在程序中使用maxn时,可以代替100010进行操作,避免了代码中使用了许多数字的情况,增加了代码的可读性和可维护性。常数还可以用于数组下标、循环次数等需要明确值的地方,保证了程序的正确性以及代码的健壮性。
对于数组来说,const int maxn=100010表示定义了一个大小为maxn的数组,即数组的长度为100010。此时,在读入、处理和输出数组元素时,就可以直接使用maxn代替100010,方便快捷。
总之,const int maxn=100010这个定义可以使程序中的数字具有明确的含义,同时避免了数字在程序中被重复使用的情况,提高了程序的可读性和可维护性,也保证了程序的正确性和健壮性。
阅读全文
相关推荐
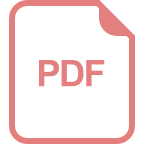
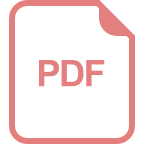
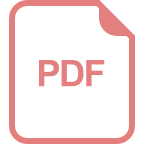













