用C语言实现电机控制的FOC算法模板
时间: 2024-05-27 08:12:31 浏览: 150
很高兴为您解答。以下是使用C语言实现电机控制的FOC算法模板的示例代码:
#include <stdio.h>
double abc_current[3]; // ABC 相电流
double alpha_beta_current[2]; // αβ 相电流
double theta; // 电机转子角度(弧度)
double u_alpha_beta[2]; // αβ 相电压
double u_qd[2]; // qd 轴电压
double idq_current[2]; // dq 轴电流
double iqs_reference; // q 轴电流参考值
double Kp = 0.1; // PI 控制器比例系数
double Ki = 0.01; // PI 控制器积分系数
double integrator = 0.0; // PI 控制器积分器
double current_limit = 10.0; // 电流限制
void abc_to_alpha_beta(double abc[3], double alpha_beta[2]) {
alpha_beta[0] = 2.0/3.0 * (abc[0] - 0.5*abc[1] - 0.5*abc[2]);
alpha_beta[1] = 2.0/3.0 * (0.866*abc[1] - 0.866*abc[2]);
}
void alpha_beta_to_dq(double alpha_beta[2], double theta, double dq[2]) {
dq[0] = alpha_beta[0] * cos(theta) + alpha_beta[1] * sin(theta);
dq[1] = -alpha_beta[0] * sin(theta) + alpha_beta[1] * cos(theta);
}
void dq_to_alpha_beta(double dq[2], double theta, double alpha_beta[2]) {
alpha_beta[0] = dq[0] * cos(theta) - dq[1] * sin(theta);
alpha_beta[1] = dq[0] * sin(theta) + dq[1] * cos(theta);
}
void pi_controller(double reference, double measured, double *output, double Kp, double Ki, double *integrator, double limit) {
double error = reference - measured;
*integrator += Ki * error;
if (*integrator > limit) {
*integrator = limit;
} else if (*integrator < -limit) {
*integrator = -limit;
}
*output = Kp * error + *integrator;
if (*output > limit) {
*output = limit;
} else if (*output < -limit) {
*output = -limit;
}
}
void current_controller(double iqs_reference, double idq[2], double u_qd[2]) {
double iqs = idq[1];
double iqs_error = iqs_reference - iqs;
pi_controller(iqs_reference, iqs, &u_qd[1], Kp, Ki, &integrator, current_limit);
u_qd[0] = idq[0];
}
void torque_controller(double torque_reference, double theta, double u_alpha_beta[2]) {
double alpha_beta[2];
alpha_beta_to_dq(alpha_beta_current, theta, idq_current);
double iqs = idq_current[1];
double iqs_error = torque_reference / (2.0/3.0 * sqrt(3.0)) - iqs;
pi_controller(torque_reference / (2.0/3.0 * sqrt(3.0)), iqs, &u_alpha_beta[1], Kp, Ki, &integrator, current_limit);
dq_to_alpha_beta(u_qd, theta, u_alpha_beta);
}
int main() {
// 读取 ABC 相电流
printf("请输入 ABC 相电流:\n");
for (int i = 0; i < 3; i++) {
scanf("%lf", &abc_current[i]);
}
// 转换为 αβ 相电流
abc_to_alpha_beta(abc_current, alpha_beta_current);
// 计算电机转子角度
theta += 0.01; // 假设每次循环转子转动 0.01 弧度
if (theta > 2.0*3.1415926) {
theta -= 2.0*3.1415926;
}
// 控制算法
iqs_reference = 5.0; // 设定 q 轴电流参考值
current_controller(iqs_reference, idq_current, u_qd);
torque_controller(0.1, theta, u_alpha_beta); // 设定转矩参考值为 0.1 Nm
// 输出 αβ 相电压
printf("αβ 相电压(V):%.2lf, %.2lf\n", u_alpha_beta[0], u_alpha_beta[1]);
return 0;
}
希望能对您有所帮助!
阅读全文
相关推荐
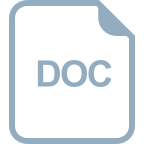
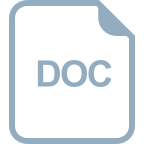
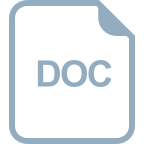
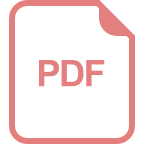


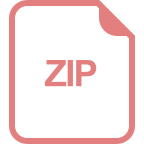
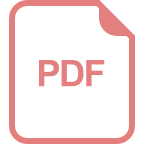
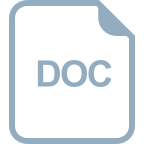
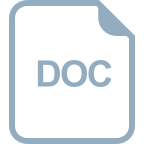
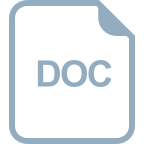
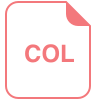
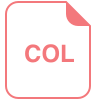
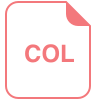
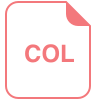



