用C++写一个棱形继承的实例
时间: 2023-09-19 21:07:05 浏览: 44
好的,以下是一个 C++ 的棱形继承实例:
```c++
#include <iostream>
using namespace std;
// 基类 Shape
class Shape {
public:
void setWidth(int w) {
width = w;
}
void setHeight(int h) {
height = h;
}
protected:
int width;
int height;
};
// 第一个派生类 PaintCost
class PaintCost {
public:
int getCost(int area) {
return area * 70;
}
};
// 第二个派生类 Rectangle
class Rectangle: public Shape, public PaintCost {
public:
int getArea() {
return (width * height);
}
};
// 第三个派生类 Triangle
class Triangle: public Shape {
public:
int getArea() {
return (width * height)/2;
}
};
int main() {
Rectangle Rect;
Triangle Tri;
Rect.setWidth(5);
Rect.setHeight(7);
// 输出矩形的面积
cout << "Total area: " << Rect.getArea() << endl;
// 输出矩形的涂料费用
cout << "Total paint cost: $" << Rect.getCost(Rect.getArea()) << endl;
Tri.setWidth(5);
Tri.setHeight(7);
// 输出三角形的面积
cout << "Total triangle area: " << Tri.getArea() << endl;
return 0;
}
```
在这个例子中,我们定义了一个 `Shape` 基类,其中包含了设置宽度和高度的方法。然后,我们定义了一个 `PaintCost` 派生类,其中包含了计算涂料费用的方法。接着,我们定义了一个 `Rectangle` 派生类,它继承了 `Shape` 和 `PaintCost` 类,其中包含了计算矩形面积的方法。最后,我们定义了一个 `Triangle` 派生类,它继承了 `Shape` 类,其中包含了计算三角形面积的方法。
在 `main` 函数中,我们创建了一个 `Rectangle` 对象和一个 `Triangle` 对象,然后分别设置它们的宽度和高度,并打印出它们的面积。同时,我们还调用了 `Rectangle` 对象的 `getCost` 方法,计算出了涂料费用。
相关推荐
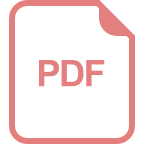







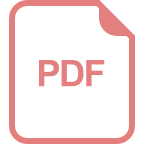