arduino esp32环境 String以空格分割成String*数组
时间: 2024-04-09 16:32:43 浏览: 154
你可以使用`String`类的`split()`函数将一个字符串以空格分割成一个`String`类型的数组。以下是一个示例代码:
```c++
String str = "Hello world! This is an example.";
int size = 0;
// 使用split()函数分割字符串
String* strArray = split(str, ' ', &size);
// 打印分割后的字符串数组
for (int i = 0; i < size; i++) {
Serial.println(strArray[i]);
}
// 释放内存
delete[] strArray;
```
这段代码将会输出以下内容:
```
Hello
world!
This
is
an
example.
```
注意,在使用完数组后,需要使用`delete[]`操作符释放内存,以避免内存泄漏。
相关问题
arduino esp32环境 String查找空格的出现次数
在 Arduino ESP32 环境中,可以使用 `String` 类的 `indexOf()` 方法和循环来查找空格的出现次数。以下是一个示例代码:
```cpp
void setup() {
Serial.begin(9600);
String myString = "Hello world! This is an example.";
int spaceCount = 0;
int index = 0;
while (index != -1) {
index = myString.indexOf(' ', index);
if (index != -1) {
spaceCount++;
index++;
}
}
Serial.print("Number of spaces: ");
Serial.println(spaceCount);
}
void loop() {
// 空函数
}
```
这个示例代码中,我们定义了一个 `String` 对象 `myString`,然后使用 `indexOf()` 方法在字符串中查找空格。如果找到空格,则计数器 `spaceCount` 加一,并将索引 `index` 加一,继续查找下一个空格。循环会一直执行直到没有找到更多的空格。最后,通过串口打印出空格的出现次数。
记得在 Arduino IDE 中选择 ESP32 开发板,然后上传并打开串口监视器,以查看输出结果。
arduino esp32 IPAddress 转String
要将Arduino ESP32的IPAddress转换为字符串,可以使用toString()方法。首先,您需要获取ESP32的本地IP地址,可以使用WiFi.localIP()函数来实现。然后,将返回的IPAddress对象使用toString()方法转换为字符串,并使用c_str()函数获取其const char*指针。最后,可以将const char*指针转换为std::string类型。
以下是一个示例代码:
```cpp
#include <WiFi.h>
void setup() {
Serial.begin(9600);
delay(1000);
// 连接WiFi网络
WiFi.begin("your_SSID", "your_password");
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
// 获取本地IP地址并转换为字符串
IPAddress localIP = WiFi.localIP();
String ipString = localIP.toString();
const char* ipChar = ipString.c_str();
// 打印转换后的IP地址字符串
Serial.print("Local IP Address: ");
Serial.println(ipChar);
}
void loop() {
// 程序的其他部分
}
```
这样,您就可以将ESP32的本地IP地址转换为字符串并打印出来了。
阅读全文
相关推荐
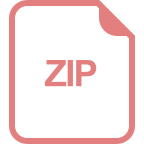
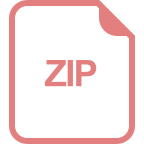













