请求一个用C语言实现的KMP算法(Knuth-Morris-Pratt)来处理数据串匹配的详细代码示例
时间: 2024-10-29 07:11:56 浏览: 29
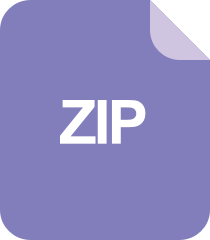
KMP-knuth-morris-pratt-Python:在文本中找到模式的Knuth-Morris-Pratt算法的实现
在C语言中,KMP(Knuth-Morris-Preparat)算法用于在文本字符串中查找模式串的位置。这是一种高效的字符串搜索算法,通过预计算部分匹配表(也称为失配函数),避免了不必要的字符比较。以下是详细的C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
// 创建失配函数数组
int getMismatchTable(char pattern[], int n) {
int mismatch[] = {0};
int j = -1;
for (int i = 1; i < n; ++i) {
while (j != -1 && pattern[j + 1] != pattern[i]) {
j = mismatch[j];
}
if (pattern[j + 1] == pattern[i]) {
j++;
}
mismatch[i] = j;
}
return mismatch;
}
// KMP搜索函数
void kmpSearch(char text[], char pattern[], int n) {
int i = 0, j = 0;
int mismatch[] = getMismatchTable(pattern, n);
while (i < strlen(text)) {
if (text[i] == pattern[j]) {
i++, j++;
} else if (j != 0) {
j = mismatch[j - 1];
} else {
i++;
}
if (j == n) {
printf("Pattern found at index %d\n", i - j);
j = mismatch[j - 1]; // 如果找到模式,跳回失配位置继续搜寻
}
}
}
int main() {
char text[] = "ABABCABABCC";
char pattern[] = "ABC";
int patternLength = strlen(pattern);
kmpSearch(text, pattern, patternLength);
return 0;
}
```
在这个代码中,`getMismatchTable`函数创建了一个失配函数数组,`kmpSearch`函数则是实际执行KMP匹配的过程。当遇到不匹配的情况时,会根据失配函数调整模式串的指针。
阅读全文
相关推荐
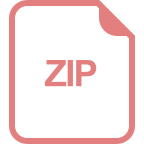
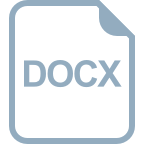
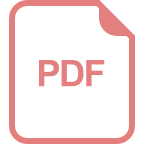
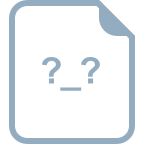
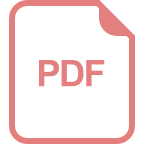
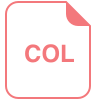

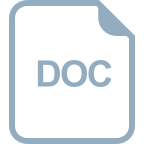

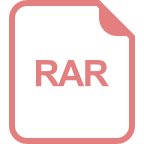
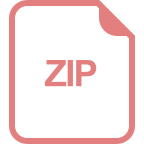
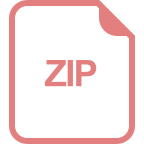
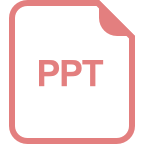
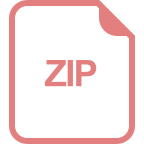
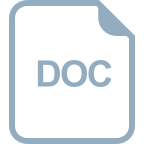
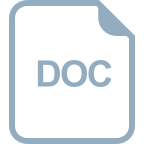
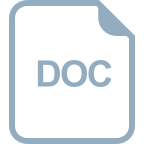
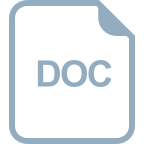
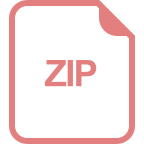