安卓开发使用高德地图获取当前定位的5公里范围并显示出来,请用kotlin实现
时间: 2024-05-04 07:18:10 浏览: 16
以下是使用 Kotlin 实现获取当前位置的 5 公里范围内的地点并在地图上显示的示例代码:
1. 添加依赖
在你的 `build.gradle` 文件中添加以下依赖:
```gradle
dependencies {
implementation 'com.amap.api:maps:5.5.0'
implementation 'com.amap.api:location:5.4.0'
}
```
2. 获取权限
在 `AndroidManifest.xml` 文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
```
3. 配置地图
在布局文件中添加 `MapView` 控件:
```xml
<com.amap.api.maps.MapView
android:id="@+id/mapView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
在 Activity 或 Fragment 中获取 `MapView` 控件并配置地图参数:
```kotlin
class MainActivity : AppCompatActivity(), AMapLocationListener {
private lateinit var mapView: MapView
private lateinit var aMap: AMap
private lateinit var locationClient: AMapLocationClient
private lateinit var locationOption: AMapLocationClientOption
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
mapView = findViewById(R.id.mapView)
mapView.onCreate(savedInstanceState)
aMap = mapView.map
aMap.uiSettings.isZoomControlsEnabled = true
locationOption = AMapLocationClientOption()
locationOption.locationMode = AMapLocationClientOption.AMapLocationMode.Hight_Accuracy
locationOption.isOnceLocation = true
locationClient = AMapLocationClient(this)
locationClient.setLocationOption(locationOption)
locationClient.setLocationListener(this)
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if (checkSelfPermission(Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
requestPermissions(arrayOf(Manifest.permission.ACCESS_FINE_LOCATION), 1)
} else {
locationClient.startLocation()
}
} else {
locationClient.startLocation()
}
}
override fun onRequestPermissionsResult(requestCode: Int, permissions: Array<String>, grantResults: IntArray) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults)
if (requestCode == 1 && grantResults.isNotEmpty() && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
locationClient.startLocation()
}
}
override fun onLocationChanged(location: AMapLocation?) {
if (location != null) {
if (location.errorCode == 0) {
val latLng = LatLng(location.latitude, location.longitude)
val cameraUpdate = CameraUpdateFactory.newLatLngZoom(latLng, 15f)
aMap.moveCamera(cameraUpdate)
val search = PoiSearch(this, PoiSearch.Query("", "", location.cityCode))
search.bound = PoiSearch.SearchBound(latLng, 5000)
search.setOnPoiSearchListener(object: PoiSearch.OnPoiSearchListener {
override fun onPoiSearched(result: PoiResult?, code: Int) {
if (code == AMapException.CODE_AMAP_SUCCESS && result != null) {
for (poiItem in result.pois) {
val markerOptions = MarkerOptions()
markerOptions.position(poiItem.latLonPoint.toLatLng())
markerOptions.title(poiItem.title)
markerOptions.snippet(poiItem.snippet)
aMap.addMarker(markerOptions)
}
}
}
override fun onPoiItemSearched(p0: PoiItem?, p1: Int) {}
})
search.searchPOIAsyn()
} else {
Toast.makeText(this, "定位失败", Toast.LENGTH_SHORT).show()
}
} else {
Toast.makeText(this, "定位失败", Toast.LENGTH_SHORT).show()
}
}
override fun onResume() {
super.onResume()
mapView.onResume()
}
override fun onPause() {
super.onPause()
mapView.onPause()
}
override fun onSaveInstanceState(outState: Bundle) {
super.onSaveInstanceState(outState)
mapView.onSaveInstanceState(outState)
}
override fun onDestroy() {
super.onDestroy()
mapView.onDestroy()
}
}
fun LatLonPoint.toLatLng(): LatLng {
return LatLng(latitude, longitude)
}
```
在 `onLocationChanged` 回调中,我们使用高德地图的 `PoiSearch` 类来搜索当前位置 5 公里范围内的地点,并在地图上添加标记。注意,这里我们使用了 Kotlin 扩展函数 `LatLonPoint.toLatLng()` 将 `LatLonPoint` 转换为 `LatLng`。
这样,当用户打开应用时,我们会请求定位权限并开始定位。当定位成功后,我们将地图中心移到当前位置,并显示 5 公里范围内的地点。
相关推荐
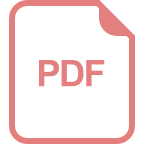














