用easyx写一个贪吃蛇游戏
时间: 2023-07-01 09:07:10 浏览: 94
好的,下面是用EasyX库写的贪吃蛇游戏代码:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
const int WIDTH = 800; // 窗口宽度
const int HEIGHT = 600; // 窗口高度
const int CELL_SIZE = 20; // 格子大小
const int ROWS = HEIGHT / CELL_SIZE; // 行数
const int COLS = WIDTH / CELL_SIZE; // 列数
const int MAX_LENGTH = ROWS * COLS; // 蛇的最大长度
enum Direction { UP, DOWN, LEFT, RIGHT }; // 方向枚举
struct Point {
int x, y;
Point(int x = 0, int y = 0) : x(x), y(y) {}
};
class Snake {
public:
Snake(Point head = Point(COLS / 2, ROWS / 2));
void move(Direction dir);
bool isDead() const;
bool isEatFood(const Point& foodPos) const;
void grow();
void draw() const;
private:
Point body[MAX_LENGTH];
int length;
};
Snake::Snake(Point head) {
body[0] = head;
length = 1;
}
void Snake::move(Direction dir) {
for (int i = length - 1; i > 0; --i) {
body[i] = body[i - 1];
}
switch (dir) {
case UP:
--body[0].y;
break;
case DOWN:
++body[0].y;
break;
case LEFT:
--body[0].x;
break;
case RIGHT:
++body[0].x;
break;
}
}
bool Snake::isDead() const {
if (body[0].x < 0 || body[0].x >= COLS || body[0].y < 0 || body[0].y >= ROWS) {
return true;
}
for (int i = 1; i < length; ++i) {
if (body[i].x == body[0].x && body[i].y == body[0].y) {
return true;
}
}
return false;
}
bool Snake::isEatFood(const Point& foodPos) const {
return body[0].x == foodPos.x && body[0].y == foodPos.y;
}
void Snake::grow() {
body[length++] = body[length - 1];
}
void Snake::draw() const {
setfillcolor(GREEN);
setlinecolor(WHITE);
for (int i = 0; i < length; ++i) {
fillrectangle(body[i].x * CELL_SIZE, body[i].y * CELL_SIZE,
(body[i].x + 1) * CELL_SIZE, (body[i].y + 1) * CELL_SIZE);
rectangle(body[i].x * CELL_SIZE, body[i].y * CELL_SIZE,
(body[i].x + 1) * CELL_SIZE, (body[i].y + 1) * CELL_SIZE);
}
}
int main() {
initgraph(WIDTH, HEIGHT);
setbkcolor(BLACK);
settextcolor(WHITE);
settextstyle(20, 0, _T("Consolas"));
srand(static_cast<unsigned int>(time(nullptr)));
Snake snake;
Point foodPos(rand() % COLS, rand() % ROWS);
Direction dir = RIGHT;
while (!snake.isDead()) {
if (kbhit()) {
char ch = _getch();
switch (ch) {
case 'w':
case 'W':
if (dir != DOWN) {
dir = UP;
}
break;
case 's':
case 'S':
if (dir != UP) {
dir = DOWN;
}
break;
case 'a':
case 'A':
if (dir != RIGHT) {
dir = LEFT;
}
break;
case 'd':
case 'D':
if (dir != LEFT) {
dir = RIGHT;
}
break;
}
}
cleardevice();
if (snake.isEatFood(foodPos)) {
snake.grow();
foodPos = Point(rand() % COLS, rand() % ROWS);
}
snake.move(dir);
snake.draw();
setfillcolor(RED);
setlinecolor(WHITE);
fillrectangle(foodPos.x * CELL_SIZE, foodPos.y * CELL_SIZE,
(foodPos.x + 1) * CELL_SIZE, (foodPos.y + 1) * CELL_SIZE);
rectangle(foodPos.x * CELL_SIZE, foodPos.y * CELL_SIZE,
(foodPos.x + 1) * CELL_SIZE, (foodPos.y + 1) * CELL_SIZE);
_stprintf_s(_T("Score: %d"), snake.length - 1);
outtextxy(10, 10, _T("Score: 0"));
Sleep(100);
}
_stprintf_s(_T("Game over! Your score is %d"), snake.length - 1);
outtextxy(WIDTH / 2 - 120, HEIGHT / 2, _T("Game over! Your score is 0"));
_getch();
closegraph();
return 0;
}
```
编译运行后,就可以开始玩贪吃蛇游戏了。使用 W、S、A、D 或方向键控制蛇的移动方向,吃到食物会增加蛇的长度,撞到墙壁或自己的身体就会游戏结束。
阅读全文
相关推荐
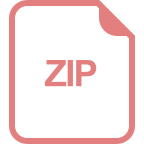





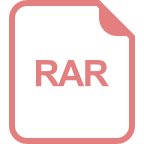
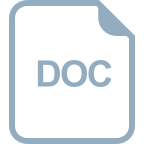
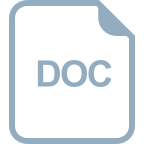
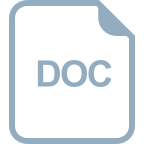

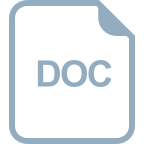

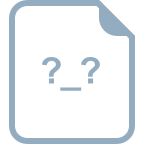