case BLE_GAP_EVT_DISCONNECTED: { m_conn_handle = BLE_CONN_HANDLE_INVALID; break; } case BLE_GATTC_EVT_PRIM_SRVC_DISC_RSP: { ble_gattc_evt_prim_srvc_disc_rsp_t *p_response = &p_ble_evt->evt.gattc_evt.params.prim_srvc_disc_rsp; // Traverse all discovered services for (uint32_t i = 0; i < p_response->count; i++) { ble_uuid_t uuid = p_response->services[i].uuid; // Determine the service type based on the UUID if (ble_uuid_cmp(&uuid, &m_svc_uuid) == 0) { // Save the service handle m_svc_handle = p_response->services[i].handle_range.start_handle; } } break; } case BLE_GATTC_EVT_CHAR_DISC_RSP: { ble_gattc_evt_char_disc_rsp_t *p_response = &p_ble_evt->evt.gattc_evt.params.char_disc_rsp; // Traverse all discovered characteristics for (uint32_t i = 0; i < p_response->count; i++) { ble_uuid_t uuid = p_response->chars[i].uuid; // Determine the characteristic type based on the UUID if (ble_uuid_cmp(&uuid, &m_char_uuid) == 0) { // Save the characteristic handle m_char_handle = p_response->chars[i].handle_value; } } // Characteristic discovery completed, can perform read/write operations, etc. break; }
时间: 2024-02-14 10:23:47 浏览: 35
这段代码是关于 BLE(蓝牙低功耗)连接和服务/特征发现的处理。在第一个 case 中,处理 BLE 设备断开连接的事件。在第二个 case 中,处理主服务发现响应事件。代码通过遍历发现的服务列表,用 UUID 来确定服务类型,并保存服务句柄。在第三个 case 中,处理特征发现响应事件。代码通过遍历发现的特征列表,用 UUID 来确定特征类型,并保存特征句柄。在这个 case 结束时,特征发现已经完成,可以执行读/写等操作。
相关问题
为什么是在这个case BLE_GAP_EVT_ADV_REPORT:情况下判断UUID是否相同呢
在Bluetooth Low Energy(BLE)中,设备可以广播包含特定UUID的广播数据。当一个BLE设备处于扫描模式时,它将收到来自其他设备的广播报告,这些广播报告中包含广播设备的UUID。
在BLE_GAP_EVT_ADV_REPORT事件中,应用程序可以获取到接收到的广播报告,并且可以从广播报告中获取广播设备的UUID。因此,在这个事件中判断UUID是否相同,是为了判断接收到的广播报告是否来自目标设备。如果UUID相同,说明接收到的广播报告来自目标设备,可以执行后续操作。如果UUID不同,说明接收到的广播报告不是目标设备的,可以忽略该广播报告。
static void ble_evt_handler(ble_evt_t const * p_ble_evt, void * p_context) { uint16_t conn_handle = p_ble_evt->evt.gap_evt.conn_handle; uint16_t role = ble_conn_state_role(conn_handle); // Based on the role this device plays in the connection, dispatch to the right handler. if (role == BLE_GAP_ROLE_PERIPH || ble_evt_is_advertising_timeout(p_ble_evt)) { ble_evt_dispatch(p_ble_evt, p_context); } else if ((role == BLE_GAP_ROLE_CENTRAL) || (p_ble_evt->header.evt_id == BLE_GAP_EVT_ADV_REPORT)) { ble_module_central_evt(p_ble_evt, p_context); } }
这是一个 Bluetooth Low Energy (BLE) 事件处理函数,用于处理 BLE 事件。它首先从事件中获取连接句柄和连接角色信息,然后根据角色信息将事件分发给不同的处理函数。如果设备角色是 BLE_GAP_ROLE_PERIPH,或者事件是广告超时事件,则将事件分发给 ble_evt_dispatch 函数进行处理。如果设备角色是 BLE_GAP_ROLE_CENTRAL,或者事件是 BLE_GAP_EVT_ADV_REPORT,则将事件分发给 ble_module_central_evt 函数进行处理。
相关推荐
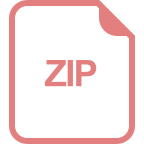












