href发送post请求
时间: 2023-08-26 13:12:16 浏览: 42
`href` 属性本身并不能直接发送 POST 请求,因为它是一个 HTML 属性,仅用于指定链接的目标 URL。但是,你可以使用 JavaScript 来实现在点击链接时发送 POST 请求。
一种实现方式是使用 `XMLHttpRequest` 对象来发送 POST 请求,例如:
```
<a href="#" onclick="sendPostRequest()">Send POST Request</a>
<script>
function sendPostRequest() {
var xhr = new XMLHttpRequest();
xhr.open("POST", "http://www.example.com", true);
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
xhr.send("name=value");
}
</script>
```
在这个例子中,我们通过在链接上绑定 `sendPostRequest()` 函数,在函数中使用 `XMLHttpRequest` 对象发送 POST 请求。需要注意的是,我们在请求头中设置了 `Content-Type` 为 `application/x-www-form-urlencoded`,并且在 `send()` 方法中传递了请求参数 `"name=value"`。
另一种实现方式是使用 `fetch` API,例如:
```
<a href="#" onclick="sendPostRequest()">Send POST Request</a>
<script>
function sendPostRequest() {
fetch("http://www.example.com", {
method: "POST",
headers: {
"Content-Type": "application/x-www-form-urlencoded"
},
body: "name=value"
})
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error(error));
}
</script>
```
在这个例子中,我们使用 `fetch` API 发送 POST 请求,并且在请求头中设置了 `Content-Type`,在 `body` 中传递了请求参数 `"name=value"`。需要注意的是,在使用 `fetch` API 发送 POST 请求时,需要使用 `then` 方法和 `catch` 方法来处理响应结果和错误。
相关推荐
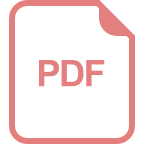














