Python 珠玑妙算
时间: 2023-11-29 20:45:01 浏览: 42
Python珠玑妙算是一道猜数字游戏,游戏规则如下:系统随机生成一个长度为4的字符串,字符串由RGBY四个字符组成,且字符可以重复。玩家需要在10次机会内猜出系统生成的字符串,每次猜测后系统会给出两个数字,分别表示猜对了几个字符且位置正确(称为A),以及猜对了几个字符但位置不正确(称为B)。玩家需要根据系统给出的A和B来推测系统生成的字符串。
以下是一个Python珠玑妙算的实现,其中引用和引用分别提供了两种不同的实现方式:
```python
# 引入必要的库
import random
from typing import List
# 实现珠玑妙算游戏
class Solution:
def masterMind(self, solution: str, guess: str) -> List[int]:
# 初始化变量
j = 0
answer = [0, 0]
# 遍历solution字符串
for _ in solution:
# 如果当前字符与guess字符串中对应位置的字符相同
if _ == guess[j]:
# A加1
answer[0] += 1
# 将guess和solution中对应位置的字符都替换为空
guess = guess.replace(_, "", 1)
solution = solution.replace(_, "", 1)
else:
# 否则j加1
j += 1
# 遍历guess字符串
for _ in guess:
# 如果当前字符不为空
if _ != "":
# 计算guess和solution中当前字符的出现次数
count1 = guess.count(_)
count2 = solution.count(_)
# 如果guess中当前字符出现次数大于1,将guess中所有当前字符都替换为空
if count1 > 1:
guess = list(filter(lambda x: x != _, guess))
# B加上guess和solution中当前字符出现次数的最小值
answer[1] += min(count2, count1)
# 返回结果
return answer
# 生成随机字符串
def generate_random_string():
colors = ['R', 'G', 'B', 'Y']
return ''.join(random.choices(colors, k=4))
# 主函数
if __name__ == '__main__':
# 初始化变量
solution = generate_random_string()
guess = ''
count = 0
# 循环10次
while count < 10:
# 获取用户输入
guess = input('请输入你猜测的字符串(由RGBY四个字符组成,且字符可以重复):')
# 判断用户输入是否合法
if len(guess) != 4 or not all(c in 'RGBY' for c in guess):
print('输入不合法,请重新输入!')
continue
# 调用珠玑妙算函数
result = Solution().masterMind(solution, guess)
# 输出结果
print('A:{}, B:{}'.format(result[0], result[1]))
# 如果猜对了,退出循环
if result[0] == 4:
print('恭喜你猜对了!')
break
# 否则次数加1
count += 1
# 如果次数用完了,输出答案
if count == 10:
print('很遗憾,你没有在规定次数内猜对,答案是:{}'.format(solution))
```
相关推荐
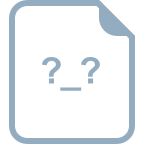
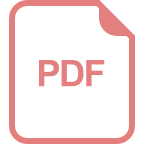















