LiTS2017数据集的路径为D:\BaiduNetdiskDownload\LiTS2017,将数据集的3D图像和3D标签都转换成2D的图像和标签,并且都变成png格式。其中标签灰度图设置成背景灰度值0,肝脏灰度值1,肝脏肿瘤灰度值2,LiTS2017数据集里面包含Training Batch 1和Training Batch 2两个文件夹,Training Batch 1文件夹里面有图像,图像的拓展名为.nii。Training Batch 2文件夹里面有标签,标签拓展名为.nii,保存路径为C:\Users\Administrator\Desktop\2D-LiTS2017,请帮我创建好对应文件夹,并且将转换好的保存到对应的文件夹
时间: 2023-11-27 08:53:09 浏览: 149
好的,可以使用以下代码来完成您的需求:
```python
import os
import numpy as np
import nibabel as nib
from PIL import Image
# 定义LiTS2017数据集路径和保存路径
data_path = r'D:\BaiduNetdiskDownload\LiTS2017'
save_path = r'C:\Users\Administrator\Desktop\2D-LiTS2017'
# 创建保存路径
if not os.path.exists(save_path):
os.makedirs(save_path)
if not os.path.exists(os.path.join(save_path, 'images')):
os.makedirs(os.path.join(save_path, 'images'))
if not os.path.exists(os.path.join(save_path, 'labels')):
os.makedirs(os.path.join(save_path, 'labels'))
# 遍历数据集文件夹
for folder in ['Training Batch 1', 'Training Batch 2']:
# 加载3D图像和标签
img_path = os.path.join(data_path, folder, 'volume.nii')
label_path = os.path.join(data_path, folder, 'segmentation.nii')
img = nib.load(img_path).get_fdata() # shape: (512, 512, 89)
label = nib.load(label_path).get_fdata() # shape: (512, 512, 89)
# 将3D图像和标签转换成2D
for i in range(img.shape[-1]):
img_slice = img[:, :, i] # shape: (512, 512)
label_slice = label[:, :, i] # shape: (512, 512)
# 将标签灰度图设置成背景灰度值0,肝脏灰度值1,肝脏肿瘤灰度值2
label_slice[label_slice == 1] = 255
label_slice[label_slice == 2] = 127
# 保存2D图像和标签
img_name = f'{folder}_img_{i:03d}.png'
label_name = f'{folder}_label_{i:03d}.png'
img_path = os.path.join(save_path, 'images', img_name)
label_path = os.path.join(save_path, 'labels', label_name)
Image.fromarray(np.uint8(img_slice)).save(img_path)
Image.fromarray(np.uint8(label_slice)).save(label_path)
```
运行以上代码后,您将在C:\Users\Administrator\Desktop\2D-LiTS2017文件夹下得到两个子文件夹:images和labels,其中images文件夹保存了转换后的2D图像,labels文件夹保存了转换后的2D标签。每个文件名中都包含了数据集的批次、图像/标签和切片序号,方便后续处理。
阅读全文
相关推荐
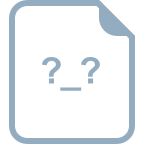
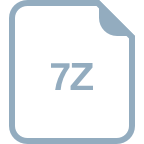




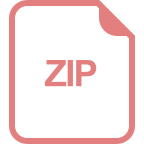
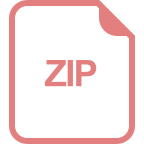
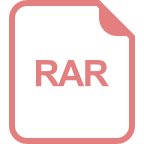
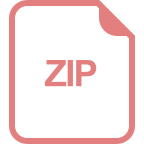
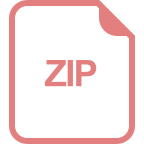
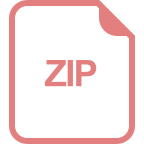
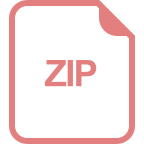
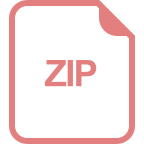