如何用java将ZonedDateTime格式转换成yyyy MM-dd
时间: 2024-04-08 18:30:04 浏览: 31
您可以使用`DateTimeFormatter`类来将`ZonedDateTime`对象转换为指定格式的日期字符串。下面是一个示例代码:
```java
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
ZonedDateTime now = ZonedDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy MM-dd");
String formattedDate = now.format(formatter);
System.out.println(formattedDate);
}
}
```
在上述代码中,我们首先创建了一个`ZonedDateTime`对象`now`,它表示当前日期和时间。然后,我们使用`DateTimeFormatter`的`ofPattern`方法创建了一个格式化模式,其中包含了"yyyy MM-dd"来定义我们想要的日期格式。最后,我们调用`now`对象的`format`方法,并将格式化模式传递给它,以获取格式化后的日期字符串。然后,我们将其打印出来。
请注意,这只是一个简单的示例,您可以根据需要调整代码来适应您的具体情况。
相关问题
java中ZonedDateTime格式如何转变为yyyy mm-dd-hh
您可以使用`DateTimeFormatter`类来将`ZonedDateTime`格式转换为您想要的格式,如下所示:
```java
import java.time.*;
import java.time.format.*;
public class Main {
public static void main(String[] args) {
ZonedDateTime zonedDateTime = ZonedDateTime.now(); // 获取当前时间
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy MM-dd-HH"); // 指定要转换的格式
String formattedDateTime = zonedDateTime.format(formatter); // 格式化时间
System.out.println(formattedDateTime);
}
}
```
上述代码中,我们首先获取当前时间的`ZonedDateTime`对象,然后创建一个`DateTimeFormatter`对象,指定要转换的格式为"yyyy MM-dd-HH"。最后,我们使用`format()`方法将`ZonedDateTime`对象格式化为指定的格式,并将结果打印出来。
请注意,格式中的字母表示不同的时间单位,例如:
- `yyyy`:四位数的年份
- `MM`:两位数的月份
- `dd`:两位数的日期
- `HH`:两位数的小时(24小时制)
您可以根据需要调整格式中的字母来满足您的要求。
如何将ZonedDateTime格式转变为yyyy-MM-dd格式 但是数据类型不变
要将`ZonedDateTime`格式转换为`yyyy-MM-dd`格式,但保持数据类型不变,您可以使用`DateTimeFormatter`类将其格式化为字符串,然后再将字符串解析回`ZonedDateTime`对象。
下面是一个示例代码:
```java
import java.time.*;
import java.time.format.*;
public class Main {
public static void main(String[] args) {
ZonedDateTime zonedDateTime = ZonedDateTime.now(); // 获取当前时间
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd"); // 指定要转换的格式
String formattedDateTime = zonedDateTime.format(formatter); // 格式化时间
ZonedDateTime parsedDateTime = ZonedDateTime.parse(formattedDateTime, formatter); // 解析为ZonedDateTime对象
System.out.println("原始时间: " + zonedDateTime);
System.out.println("格式化后的时间: " + formattedDateTime);
System.out.println("解析后的时间: " + parsedDateTime);
}
}
```
在上述代码中,我们首先获取当前时间的`ZonedDateTime`对象,然后创建一个`DateTimeFormatter`对象,指定要转换的格式为"yyyy-MM-dd"。之后,我们使用`format()`方法将`ZonedDateTime`对象格式化为指定的格式,并将结果存储在字符串变量`formattedDateTime`中。
接下来,我们使用`parse()`方法将格式化后的字符串解析回`ZonedDateTime`对象,并将结果存储在`parsedDateTime`变量中。
最后,我们分别打印原始时间、格式化后的时间和解析后的时间。
请注意,解析后的`parsedDateTime`对象与原始的`zonedDateTime`对象具有相同的数据类型,只是将格式进行了更改。
相关推荐
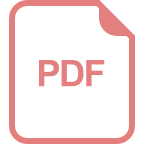













