std::string 分配内存
时间: 2024-02-20 15:55:13 浏览: 64
std::string是C++标准库中的一个类,用于表示字符串。它封装了一系列操作字符串的方法,并且自动处理内存分配和释放的细节。
当我们创建一个std::string对象时,它会自动分配足够的内存来存储字符串的内容。std::string使用动态内存分配来管理字符串的存储空间,这意味着它可以根据需要动态地增加或减少内存的使用量。
在内部实现上,std::string通常使用堆内存来存储字符串的内容。它会根据字符串的长度动态地分配足够的内存空间,并将字符串的内容复制到这块内存中。当我们对std::string对象进行赋值、拷贝或修改操作时,它会自动调整内存的大小以适应新的字符串内容。
std::string类提供了一些方法来管理内存分配,例如:
- reserve():用于预分配一定数量的内存空间,以避免频繁的内存重新分配。
- capacity():返回当前分配的内存空间大小。
- shrink_to_fit():请求std::string对象减少其容量以适应其当前大小。
总之,std::string类会自动处理字符串的内存分配和释放,使我们能够方便地操作字符串而不必担心内存管理的细节。
相关问题
std::string释放内存
在C++中,如果使用了new关键字来动态分配内存,就需要使用delete操作符来释放内存。对于std::string类型的对象,它内部的存储空间也是动态分配的,因此同样需要使用delete操作符来释放内存。但是,std::string类型的对象通常都是使用栈上分配的方式来创建的,因此不需要手动释放内存。
如果确实需要手动释放std::string类型对象的内存,可以使用delete操作符,例如:
```c++
std::string* strPtr = new std::string("example");
delete strPtr;
```
但是这种方式并不推荐,因为std::string类型的对象通常都是使用栈上分配的方式来创建的,并且C++11标准还引入了智能指针和移动语义等机制,可以更方便地管理内存。
std::string设置内存大小
在C++中,`std::string`是一个动态大小的字符串类,其内存大小由其内容决定。`std::string`会自动管理内存,所以通常不需要手动设置其内存大小。
如果你想在创建`std::string`对象时预留一定的内存空间,可以使用`reserve()`函数。`reserve()`函数用于预留足够的内存空间,以避免频繁的重新分配内存。
```cpp
std::string str;
str.reserve(100); // 预留100个字符的内存空间
```
注意,`reserve()`函数只是预留了内存空间,并没有改变`std::string`对象的实际大小。如果你想改变`std::string`对象的大小,可以使用`resize()`函数。
```cpp
std::string str;
str.resize(100); // 将字符串的大小设置为100,多出的部分用默认值填充
```
需要注意的是,以上方法只是在创建或修改`std::string`对象时设置内存大小,并不会直接修改已有对象的内存大小。`std::string`会自动根据其内容调整内存大小。
相关推荐
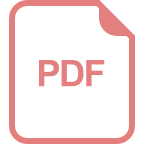
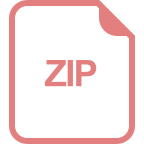
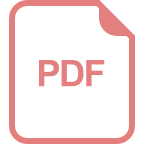
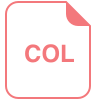
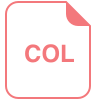
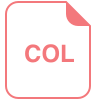
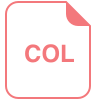








