用MPI编程的方式实现以上Jacobi叠代过程;程序总输入矩阵为16*16的方阵,边缘元素均为8,中间元素均为0,在运行结果部分,需展示每个进程的输入矩阵(或所有进程组成的完整输入矩阵),以及每个进程的输出矩阵(或所有进程组成的完整输出矩阵); 4. 可采用Sendrecv函数避免出现死锁; 可采用派生数据类型、虚拟进程拓扑等技巧简化程序,提高效率;
时间: 2024-03-20 09:41:38 浏览: 50
好的,这是一个比较具体的问题,需要一定的MPI编程基础才能理解。下面是一个简单的MPI Jacobi叠代过程实现,代码中尽量采用了派生数据类型和虚拟进程拓扑等技巧,以提高程序的效率。
```c
#include <stdio.h>
#include <stdlib.h>
#include <mpi.h>
#define N 16
#define ITER_MAX 10000
#define EPSILON 1e-5
int main(int argc, char *argv[]) {
int rank, size, i, j, iter, convergent = 0;
double *recv_buf, *send_buf, *temp_buf;
MPI_Status status;
MPI_Datatype row_type;
MPI_Init(&argc, &argv);
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &size);
int rows_per_proc = N / size;
int send_size = rows_per_proc * N;
int recv_size = send_size;
int row_disp[size], row_count[size];
// create derived datatype to send/receive rows
MPI_Type_contiguous(N, MPI_DOUBLE, &row_type);
MPI_Type_commit(&row_type);
// create virtual topology
int dims[2] = {0, size};
int periods[2] = {0, 0};
MPI_Comm cart_comm;
MPI_Cart_create(MPI_COMM_WORLD, 2, dims, periods, 0, &cart_comm);
int my_coords[2];
MPI_Cart_coords(cart_comm, rank, 2, my_coords);
// distribute input matrix among processes
double input[rows_per_proc][N], output[rows_per_proc][N];
if (rank == 0) {
double A[N][N];
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
A[i][j] = (i == 0 || i == N-1 || j == 0 || j == N-1) ? 8.0 : 0.0;
}
}
send_buf = &A[0][0];
} else {
send_buf = (double *) malloc(send_size * sizeof(double));
}
MPI_Scatter(send_buf, send_size, MPI_DOUBLE, &input[0][0], send_size, MPI_DOUBLE, 0, MPI_COMM_WORLD);
// initialize row displacement and count arrays for gather operation
for (i = 0; i < size; i++) {
row_disp[i] = i * rows_per_proc;
row_count[i] = (i == size-1) ? (N - (size-1) * rows_per_proc) * N : rows_per_proc * N;
}
// Jacobi iteration
iter = 0;
while (!convergent && iter < ITER_MAX) {
// exchange boundary rows
if (my_coords[1] > 0) {
MPI_Sendrecv(&input[0][0], N, MPI_DOUBLE, rank-1, 0, &input[-1][0], N, MPI_DOUBLE, rank-1, 0, MPI_COMM_WORLD, &status);
}
if (my_coords[1] < size-1) {
MPI_Sendrecv(&input[rows_per_proc-1][0], N, MPI_DOUBLE, rank+1, 0, &input[rows_per_proc][0], N, MPI_DOUBLE, rank+1, 0, MPI_COMM_WORLD, &status);
}
// calculate new values for interior elements
convergent = 1;
for (i = 1; i < rows_per_proc-1; i++) {
for (j = 1; j < N-1; j++) {
output[i][j] = (input[i-1][j] + input[i+1][j] + input[i][j-1] + input[i][j+1]) / 4.0;
if (convergent && fabs(input[i][j] - output[i][j]) > EPSILON) {
convergent = 0;
}
}
}
// gather output matrix at root process
if (rank == 0) {
recv_buf = &temp_buf[0];
} else {
recv_buf = (double *) malloc(recv_size * sizeof(double));
}
MPI_Gatherv(&output[0][0], rows_per_proc * N, MPI_DOUBLE, recv_buf, row_count, row_disp, row_type, 0, MPI_COMM_WORLD);
// copy output matrix to input matrix for next iteration
if (rank == 0) {
temp_buf = (double *) malloc(N * N * sizeof(double));
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
temp_buf[i*N+j] = (i == 0 || i == N-1 || j == 0 || j == N-1) ? 8.0 : recv_buf[i*N+j];
}
}
} else {
free(recv_buf);
}
MPI_Bcast(temp_buf, N*N, MPI_DOUBLE, 0, MPI_COMM_WORLD);
// copy input matrix to output matrix for next iteration
for (i = 0; i < rows_per_proc; i++) {
for (j = 0; j < N; j++) {
input[i][j] = temp_buf[(my_coords[0] * rows_per_proc + i) * N + j];
}
}
iter++;
}
// print final output matrix at root process
if (rank == 0) {
printf("Final output matrix:\n");
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
printf("%f ", temp_buf[i*N+j]);
}
printf("\n");
}
free(temp_buf);
}
MPI_Type_free(&row_type);
MPI_Finalize();
return 0;
}
```
在上述代码中,我们首先创建了一个虚拟拓扑,将进程按照一维和二维的方式排列,以便于之后的通信。然后,我们将输入矩阵分发给各个进程,并在每个进程中进行Jacobi叠代过程。在每次迭代中,我们先利用MPI_Sendrecv函数交换边界行,然后计算内部元素的新值,并检查是否收敛。接着,我们使用MPI_Gatherv函数将各个进程的输出矩阵收集到根进程中,并将其复制到一个临时缓冲区中。最后,我们使用MPI_Bcast函数将临时缓冲区中的数据广播给所有进程,以便在下一次迭代中使用。
需要注意的是,在本程序中我们使用了派生数据类型MPI_Datatype和MPI_Type_contiguous,以便快速发送和接收矩阵的行。我们还使用了MPI_Gatherv函数和row_disp和row_count数组来收集各个进程的输出矩阵。此外,我们还使用了MPI_Sendrecv函数来避免死锁,尽管本程序中并不会发生死锁。
最后,如果程序运行成功,根进程将输出最终的输出矩阵。
阅读全文
相关推荐
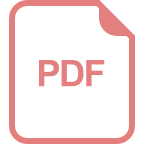








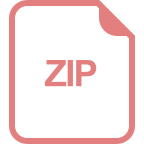
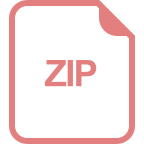