用python写一段代码,要求识别矩形,圆形,三角形,他们的颜色分别为黄色,红色,蓝色
时间: 2024-05-06 16:19:07 浏览: 17
这里给出一种基于OpenCV库的解决方案:
```python
import cv2
# 读取图像
img = cv2.imread('shapes.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 阈值化处理
thresh = cv2.threshold(gray, 100, 255, cv2.THRESH_BINARY)[1]
# 查找轮廓
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 循环遍历轮廓
for contour in contours:
# 计算轮廓周长
perimeter = cv2.arcLength(contour, True)
# 进行多边形逼近
approx = cv2.approxPolyDP(contour, 0.04 * perimeter, True)
# 获取多边形的顶点数量
vertices = len(approx)
# 获取轮廓的外接矩形
x, y, w, h = cv2.boundingRect(contour)
# 根据顶点数量判断形状
if vertices == 3: # 三角形
cv2.putText(img, 'Triangle', (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255, 0, 0), 2)
cv2.rectangle(img, (x,y), (x+w,y+h), (255, 0, 0), 2)
elif vertices == 4: # 矩形
cv2.putText(img, 'Rectangle', (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 255), 2)
cv2.rectangle(img, (x,y), (x+w,y+h), (0, 255, 255), 2)
else: # 圆形
cv2.putText(img, 'Circle', (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
cv2.circle(img, (int(x+w/2),int(y+h/2)), int(w/2), (0, 0, 255), 2)
# 显示结果
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,此代码仅适用于图像中的形状为纯色且颜色明显的情况,对于复杂背景或颜色相近的形状可能无法准确识别。
相关推荐
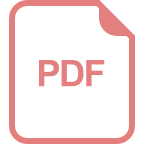
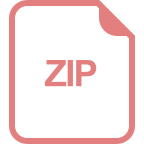














