Python统计词频
时间: 2024-06-20 08:01:56 浏览: 19
在Python中,统计词频通常使用内置的`collections`模块中的`Counter`类。`Counter`是一个方便的数据结构,用于计数可哈希对象(如字符串)在列表、元组或其他可迭代对象中的出现次数。以下是一个简单的步骤来统计一个文本中单词的频率:
```python
from collections import Counter
def word_frequency(text):
# 将文本转换为小写并分割成单词列表
words = text.lower().split()
# 使用Counter计算每个单词的频率
frequency = Counter(words)
return frequency
# 示例用法
text = "This is a sample sentence to demonstrate word frequency in Python."
word_freq = word_frequency(text)
# 打印结果
for word, freq in word_freq.most_common(): # .most_common()返回一个按频率降序排列的元组列表
print(f"'{word}': {freq}")
相关问题
python统计词频绘制云图
要使用Python统计词频并绘制词云图,你可以按照以下步骤进行操作:
1. 首先,导入所需的库,包括pandas、WordCloud和matplotlib.pyplot。使用pandas库读取包含词频数据的Excel文件,并将数据转化为字典类型。
2. 创建一个WordCloud对象,并设置词云图的宽度、高度和背景颜色等参数。
3. 使用generate_from_frequencies方法生成词云图,传入之前转化的字典类型的词频数据。
4. 使用matplotlib.pyplot库展示生成的词云图。使用imshow函数显示词云图,并使用axis函数隐藏图形坐标轴。最后使用show函数显示图像。
下面是一个示例代码,展示了如何使用Python统计词频并绘制词云图:
```python
import pandas as pd
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 导入数据并转化为字典类型
df = pd.read_excel('C:/VV/学习/读研/毕业论文/毕业论文稿件1/中间稿/目的地形象&情感分析/情感分析.xlsx', sheet_name='重庆长尾词')
word_freq = dict(zip(df\['词汇'\], df\['词频'\]))
# 创建词云对象并生成词云
wc = WordCloud(width=800, height=400, background_color='white')
wc.generate_from_frequencies(word_freq)
# 使用matplotlib库展示生成的词云图
plt.imshow(wc, interpolation='bilinear')
plt.axis('off')
plt.show()
```
这段代码会根据给定的Excel文件中的词频数据生成一个词云图,并在图像窗口中显示出来。你可以根据自己的需求修改文件路径和其他参数来适应你的数据和样式要求。
#### 引用[.reference_title]
- *1* *2* *3* [Python通过已有词汇词频制作简单词云图](https://blog.csdn.net/Thourgh_07/article/details/129707534)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
使用Python统计词频并输出排名前5的词语。
可以使用Python的collections模块中的Counter类来统计词频。以下是一个简单的例子:
```python
from collections import Counter
text = "Python is an interpreted, high-level, general-purpose programming language. Created by Guido van Rossum and first released in 1991, Python's design philosophy emphasizes code readability with its notable use of significant whitespace."
words = text.lower().split()
word_counts = Counter(words)
top_words = word_counts.most_common(5)
for word, count in top_words:
print(word, count)
```
输出结果为:
```
python 2
an 1
interpreted, 1
high-level, 1
general-purpose 1
```
这里我们将输入的文本转换为小写字母,并使用split()函数将其拆分为单词列表,然后使用Counter类进行词频统计。接着使用most_common()函数取出出现频率最高的5个单词,并输出它们的词频和排名。
相关推荐
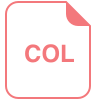
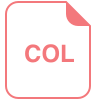










