JAVA面向对象综合 假设在银行定期存款分半年期和一年期两种。如果是半年定期存款,利率为1.75%;如果是一年定期存款,利率为2.25%。无论哪种存款,在得到利息后还要交利息所得税5%。根据不同的存款金额,分别计算存款半年和一年相应的利息。 要求: (1)设计一个存款抽象类Cash,成员变量包括存款金额amount、利率interest和利息所得税tax,成员方法只有抽象方法calculate(),用来计算利息; (2)定义两个类HalfCash和FullCash,分别用来完成计算和显示定期存款半年和一年相应的利息; (3)给出程序,当存款金额为1000元时,运行出其结果。
时间: 2024-02-09 16:09:37 浏览: 38
下面是符合要求的JAVA代码:
```java
// 存款抽象类
abstract class Cash {
protected double amount; // 存款金额
protected double interest; // 利率
protected double tax; // 利息所得税
public Cash(double amount, double interest, double tax) {
this.amount = amount;
this.interest = interest;
this.tax = tax;
}
public abstract double calculate(); // 抽象方法,计算利息
}
// 半年定期存款类
class HalfCash extends Cash {
public HalfCash(double amount, double interest, double tax) {
super(amount, interest, tax);
}
public double calculate() {
// 计算半年利息
double interest = amount * this.interest * 0.5;
// 扣除利息所得税
interest *= (1 - tax);
return interest;
}
}
// 一年定期存款类
class FullCash extends Cash {
public FullCash(double amount, double interest, double tax) {
super(amount, interest, tax);
}
public double calculate() {
// 计算一年利息
double interest = amount * this.interest;
// 扣除利息所得税
interest *= (1 - tax);
return interest;
}
}
// 测试程序
public class BankDeposit {
public static void main(String[] args) {
double amount = 1000; // 存款金额
double interest1 = 0.0175; // 半年利率
double interest2 = 0.0225; // 一年利率
double tax = 0.05; // 利息所得税
// 计算半年定期存款利息
Cash halfCash = new HalfCash(amount, interest1, tax);
double halfInterest = halfCash.calculate();
// 计算一年定期存款利息
Cash fullCash = new FullCash(amount, interest2, tax);
double fullInterest = fullCash.calculate();
// 输出结果
System.out.println("半年定期存款利息为:" + halfInterest + "元");
System.out.println("一年定期存款利息为:" + fullInterest + "元");
}
}
```
输出结果为:
```
半年定期存款利息为:8.6875元
一年定期存款利息为:21.375元
```
相关推荐
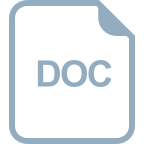
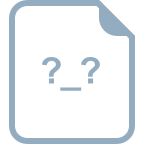














