三个线程 第一个获取输入,统计奇偶数个数,满足3个,唤醒2,3线程 第二个输出3个偶数 第三个输出3个奇数,使用条件变量
时间: 2023-08-04 22:16:05 浏览: 76
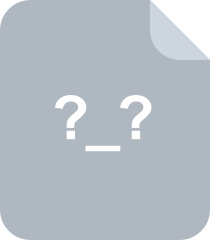
C++11 使用条件变量控制三个线程按顺序输出数字
好的,这是一个比较典型的线程同步问题,可以使用条件变量和互斥锁来实现。下面是一个可能的实现:
```c++
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
using namespace std;
mutex mtx;
condition_variable cv;
int count_even = 0;
int count_odd = 0;
void input_thread()
{
while (true)
{
int num;
cin >> num;
unique_lock<mutex> ul(mtx);
if (num % 2 == 0)
{
++count_even;
if (count_even == 3)
{
count_even = 0;
cv.notify_all();
}
}
else
{
++count_odd;
if (count_odd == 3)
{
count_odd = 0;
cv.notify_all();
}
}
}
}
void output_even_thread()
{
while (true)
{
unique_lock<mutex> ul(mtx);
cv.wait(ul, []{ return count_even == 3; });
for (int i = 0; i < 3; ++i)
{
cout << i * 2 << " ";
}
cout << endl;
}
}
void output_odd_thread()
{
while (true)
{
unique_lock<mutex> ul(mtx);
cv.wait(ul, []{ return count_odd == 3; });
for (int i = 0; i < 3; ++i)
{
cout << i * 2 + 1 << " ";
}
cout << endl;
}
}
int main()
{
thread t_input(input_thread);
thread t_output_even(output_even_thread);
thread t_output_odd(output_odd_thread);
t_input.join();
t_output_even.join();
t_output_odd.join();
return 0;
}
```
这里有三个线程,一个输入线程和两个输出线程,它们都共享两个计数器 `count_even` 和 `count_odd`。输入线程获取一个数字后,如果是偶数,就将 `count_even` 加 1,如果已经有 3 个偶数了,就唤醒等待偶数的输出线程;如果是奇数,则将 `count_odd` 加 1,如果已经有 3 个奇数了,就唤醒等待奇数的输出线程。
输出线程会等待条件变量 `cv`,并在满足条件时输出 3 个数字,然后继续等待下一轮。注意要在输出后将计数器清零,否则会一直等待。互斥锁 `mtx` 用于保护共享变量的访问,避免并发访问导致数据不一致。
阅读全文
相关推荐

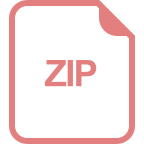
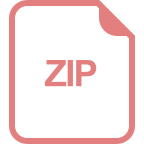
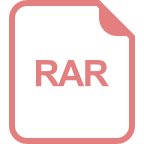
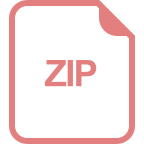
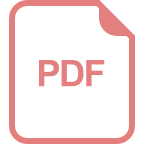
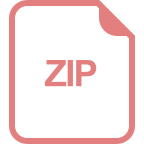
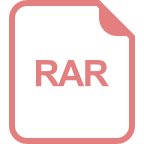
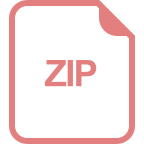
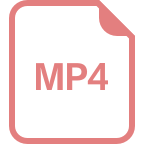
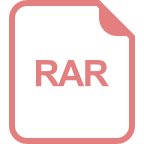
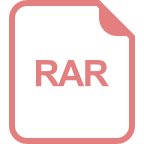
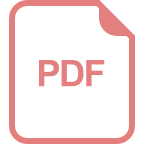
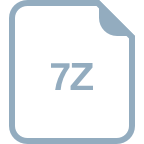
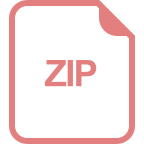