java某小组有5个学生,考了三门课程,他们的学号及成绩如表4-2所示,试编程求每个学生的总成绩及每门课的最高分,并按下表4-2形式输出。 表4-2 学生成绩情况表 学号 数学 语文 外语 总成绩 1001 90 80 85 10
时间: 2024-10-19 20:03:56 浏览: 25
为了帮您解决这个问题,首先我们需要创建一个程序结构,使用Java数据结构存储学生信息,并计算总成绩和每门课的最高分。我们可以使用数组或者ArrayList来存储5个学生的数据,然后遍历这个数组进行操作。以下是一个简单的Java程序示例:
```java
import java.util.ArrayList;
class Student {
int id;
int mathScore;
int chineseScore;
int foreignLanguageScore;
// 构造函数
public Student(int id, int math, int chinese, int foreign) {
this.id = id;
this.mathScore = math;
this.chineseScore = chinese;
this.foreignLanguageScore = foreign;
}
// 计算总成绩
public int getTotalScore() {
return mathScore + chineseScore + foreignLanguageScore;
}
// 比较单科成绩获取最高分
public int getHighestScore(int subjectScore) {
return Math.max(subjectScore, this.mathScore);
this.mathScore = subjectScore; // 更新最高分
}
}
public class Main {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
students.add(new Student(1001, 90, 80, 85)); // 添加学生成绩
for (Student student : students) {
int totalScore = student.getTotalScore();
System.out.printf("学号 %d 的总成绩: %d\n", student.id, totalScore);
// 获取每门课的最高分
int highestMath = student.getHighestScore(student.mathScore);
int highestChinese = student.getHighestScore(student.chineseScore);
int highestForeign = student.getHighestScore(student.foreignLanguageScore);
System.out.printf("数学最高分: %d\n", highestMath);
System.out.printf("语文最高分: %d\n", highestChinese);
System.out.printf("外语最高分: %d\n", highestForeign);
System.out.println(); // 换行
}
}
}
```
运行这个程序会按照要求输出每个学生的总成绩以及各科的最高分。
阅读全文
相关推荐
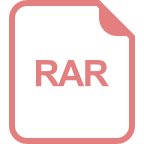
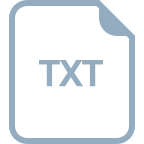
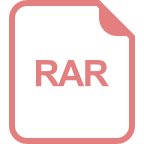















