父进程创建两个子进程,子进程1执行 server(),子进程2执行client();client发送10 条类型为10~1的消息入队列,server接收消 息。 要求:client将键盘输入的字符作为消息正文 发送出去,server只接收消息类型为5~1的消 息并打印输出消息正文内容及消息类型号 要求:父进程在等待子进程结束后删除消息 队列。
时间: 2024-02-27 12:52:24 浏览: 44
好的,这是一个关于进程间通信的问题。下面是大致的代码实现思路:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MSG_TYPE1 10
#define MSG_TYPE2 9
#define MSG_TYPE3 8
#define MSG_TYPE4 7
#define MSG_TYPE5 6
#define MSG_TYPE6 5
#define MSG_TYPE7 4
#define MSG_TYPE8 3
#define MSG_TYPE9 2
#define MSG_TYPE10 1
struct msgbuf {
long mtype;
char mtext[1024];
};
void client(int msqid) {
struct msgbuf msg;
char input[1024];
int i;
for (i = MSG_TYPE1; i <= MSG_TYPE10; i++) {
if (i >= MSG_TYPE5) {
msg.mtype = i;
printf("Please input message for type %d: ", i);
fgets(input, 1024, stdin);
strncpy(msg.mtext, input, 1024);
if (msgsnd(msqid, &msg, sizeof(msg.mtext), 0) == -1) {
perror("msgsnd");
exit(1);
}
}
}
}
void server(int msqid) {
struct msgbuf msg;
int i;
for (i = MSG_TYPE1; i <= MSG_TYPE10; i++) {
if (i >= MSG_TYPE5) {
if (msgrcv(msqid, &msg, sizeof(msg.mtext), i, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message from type %ld: %s", msg.mtype, msg.mtext);
}
}
}
int main() {
int msqid;
pid_t pid1, pid2;
key_t key;
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
if ((msqid = msgget(key, 0666 | IPC_CREAT)) == -1) {
perror("msgget");
exit(1);
}
/* 创建子进程1 */
if ((pid1 = fork()) == -1) {
perror("fork");
exit(1);
} else if (pid1 == 0) {
/* 子进程1执行server() */
server(msqid);
exit(0);
}
/* 创建子进程2 */
if ((pid2 = fork()) == -1) {
perror("fork");
exit(1);
} else if (pid2 == 0) {
/* 子进程2执行client() */
client(msqid);
exit(0);
}
/* 等待子进程1和子进程2结束 */
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
/* 删除消息队列 */
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
代码中共创建了两个子进程,分别执行 `client()` 和 `server()` 函数。其中, `client()` 函数调用 `msgsnd()` 函数将键盘输入作为消息正文发送到消息队列中, `server()` 函数调用 `msgrcv()` 函数从消息队列中接收消息,打印输出消息正文和消息类型号。父进程等待两个子进程结束后,再删除消息队列。
需要注意的是,这里使用了消息队列作为进程间通信的方式,消息队列需要使用 `msgget()` 函数创建,使用 `msgsnd()` 函数往消息队列中发送消息,使用 `msgrcv()` 函数从消息队列中接收消息。同时,还需要指定消息的类型,根据题目要求,只有消息类型为5~1的消息才会被打印输出,因此需要在发送和接收消息时进行类型的判断。
阅读全文
相关推荐
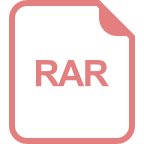
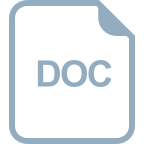
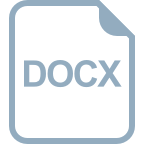














