qt怎么把动态图片当作设置为开机界面,并且做窗体自适应
时间: 2024-09-21 12:03:39 浏览: 59
在Qt中,你可以使用QPixmap和QDesktopWidget来实现动态图片作为开机界面,并且让窗体自适应屏幕。以下是一段示例代码,展示了如何做到这些:
```cpp
#include <QApplication>
#include <QPixmap>
#include <QDesktopWidget>
#include <QTimer>
class MainWindow : public QMainWindow {
public:
MainWindow(QWidget *parent = nullptr) : QMainWindow(parent) {
// 设置背景图片
QPixmap backgroundPixmap("path_to_your_dynamic_image.png"); // 替换为你的动态图片路径
QLabel *backgroundLabel = new QLabel(this);
backgroundLabel->setPixmap(backgroundPixmap);
QWidget *centralWidget = new QWidget(this);
centralWidget->setAutoFillBackground(true); // 自动填充背景色
QVBoxLayout *layout = new QVBoxLayout(centralWidget);
layout->addWidget(backgroundLabel);
setCentralWidget(centralWidget);
// 创建定时器,每隔一段时间更新图片(这里假设你的图片是循环播放的)
QTimer *timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, [this]() {
if (index_ == backgroundPixmap.numImages() - 1) {
index_ = 0;
} else {
index_++;
}
backgroundLabel->setPixmap(backgroundPixmap.copy(index_));
});
timer->start(5000); // 每5秒更新一次图片
index_ = 0; // 初始化索引
// 自适应屏幕
resize(QDesktopWidget().screenGeometry());
move(QDesktopWidget().availableGeometry().center() - rect().center());
}
private:
int index_;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
```
在这个例子中,我们创建了一个`MainWindow`类,继承自`QMainWindow`。首先设置了窗口的背景为一个`QLabel`,并用动态图片替换其默认的图标。然后创建了一个定时器,每隔5秒更新`QLabel`中的图片。
为了使窗体自适应屏幕,我们在窗口初始化时调用了`resize()`方法设置窗口大小等于屏幕的几何尺寸,然后使用`move()`方法将窗口中心移动到可用区域的中心位置。
确保你已经正确地替换了`"path_to_your_dynamic_image.png"`为你的动态图片的实际路径。如果你的图片不是循环播放的,你需要修改定时器的部分逻辑来处理图片切换。
阅读全文
相关推荐
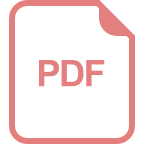
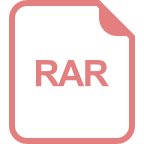
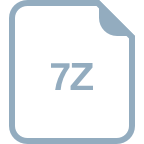

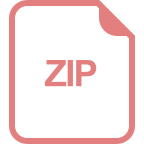
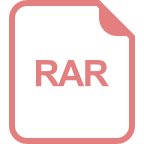
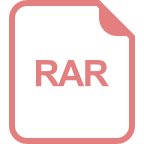










