#include<stdio.h> #include<string.h> #include<stdlib.h> int main() { char *s[80]; char *a[80]; gets(s); int i,j; j=strlen(s); for(i=0;i<j;i++) { if(s[i]>='A'&&s[i]<='Z') a[i]=s[i]+32; else a[i]=s[i]; } for(i=0;i<j;i++) { if(a[i]!=a[j-1]) { printf("no"); return 0; } } printf("yes"); return 0; } 为什么这串代码输出结果不能符合题目 判断字符是否是回文 “回文”是一种顺序读和反序读都一样的字符串,例如:“121”“abcba” “ABCCBA”编写程序,判断任一字符串是否为回文。 相关知识 通过指针来传递参数
时间: 2024-04-02 09:37:04 浏览: 10
这段代码的问题在于使用了 `gets()` 函数,这个函数容易导致缓冲区溢出,从而导致程序崩溃或输出错误结果。建议使用 `fgets()` 函数代替 `gets()` 函数,并且需要注意去掉字符串末尾的换行符。此外,还需要将小写字母转换为大写字母进行比较。修改后的代码如下:
```
#include <stdio.h>
#include <string.h>
int main() {
char s[80];
char a[80];
fgets(s, 80, stdin);
s[strcspn(s, "\n")] = '\0'; // 去掉字符串末尾的换行符
int i, j;
j = strlen(s);
for (i = 0; i < j; i++) {
if (s[i] >= 'a' && s[i] <= 'z') // 小写字母转换为大写字母
a[i] = s[i] - 32;
else
a[i] = s[i];
}
for (i = 0; i < j; i++) {
if (a[i] != a[j - 1 - i]) { // 判断是否回文
printf("no");
return 0;
}
}
printf("yes");
return 0;
}
```
相关问题
编写C语言代码,将.c文件中的#include<stdio.h>展开
以下是一个简单的C语言代码,用于将.c文件中的#include<stdio.h>展开:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void expand_header(const char* filename) {
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file!\n");
return;
}
// 创建一个新的临时文件
char* tmp_filename = strdup("tmp.c");
FILE* tmp_fp = fopen(tmp_filename, "w");
// 逐行读取输入文件
char line[1024];
while (fgets(line, sizeof(line), fp)) {
// 检查是否包含#include<stdio.h>
if (strstr(line, "#include<stdio.h>") != NULL) {
// 打开stdio.h文件并将其内容复制到临时文件中
FILE* stdio_fp = fopen("stdio.h", "r");
if (stdio_fp != NULL) {
char stdio_line[1024];
while (fgets(stdio_line, sizeof(stdio_line), stdio_fp)) {
fputs(stdio_line, tmp_fp);
}
fclose(stdio_fp);
}
} else {
// 将当前行复制到临时文件中
fputs(line, tmp_fp);
}
}
// 关闭文件并删除原来的文件
fclose(fp);
remove(filename);
// 将临时文件重命名为原来的文件名
rename(tmp_filename, filename);
// 释放内存
free(tmp_filename);
fclose(tmp_fp);
}
int main() {
const char* filename = "test.c";
expand_header(filename);
printf("Header expanded successfully!\n");
return 0;
}
```
请注意,此代码只是一个简单的示例,仅展开了一个#include<stdio.h>语句,而且还假设stdio.h文件位于当前目录中。在实际情况中,可能需要展开多个#include语句,并且可能需要处理一些特殊情况,例如条件编译指令。此外,展开头文件可能会导致代码变得冗长和难以阅读,因此在实际项目中,应该尽量避免手动展开头文件。
vs2008上,如何使用#include <tar.h> 例子
在 VS2008 中使用 `#include <tar.h>`,需要做以下几个步骤:
1. 下载 tar.h 头文件,可以从网上下载,比如在 GitHub 上可以找到该头文件的代码:https://github.com/panzi/uTAR/blob/master/tar.h
2. 将 tar.h 头文件保存在工程目录下的任意子目录中,比如 `include` 目录。
3. 在 VS2008 中打开工程,右键点击工程名称,选择“属性”,在弹出的对话框中选择“C/C++” -> “常规”,在“附加包含目录”中添加 tar.h 头文件所在目录的路径(比如 `$(ProjectDir)\include`)。
4. 在需要使用 tar.h 头文件的源文件中,加入 `#include <tar.h>` 即可。
例如,下面是一个使用 tar.h 头文件的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <tar.h>
int main(int argc, char* argv[])
{
// 创建 tar 文件
struct posix_header header;
memset(&header, 0, sizeof(header));
strncpy(header.name, "test.txt", sizeof(header.name));
strncpy(header.mode, "0000644 ", sizeof(header.mode));
strncpy(header.uid, "0000010 ", sizeof(header.uid));
strncpy(header.gid, "0000010 ", sizeof(header.gid));
strncpy(header.size, "00000000005 ", sizeof(header.size));
strncpy(header.mtime, "00000000000 ", sizeof(header.mtime));
memset(header.chksum, ' ', sizeof(header.chksum));
strncpy(header.typeflag, "0", sizeof(header.typeflag));
strncpy(header.linkname, "", sizeof(header.linkname));
strncpy(header.magic, TMAGIC, sizeof(header.magic));
strncpy(header.version, TVERSION, sizeof(header.version));
strncpy(header.uname, "", sizeof(header.uname));
strncpy(header.gname, "", sizeof(header.gname));
strncpy(header.devmajor, "", sizeof(header.devmajor));
strncpy(header.devminor, "", sizeof(header.devminor));
printf("Header checksum: %s\n", make_checksum(&header));
return 0;
}
```
相关推荐
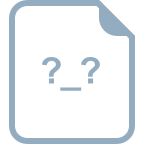
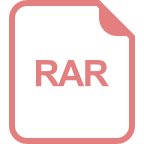
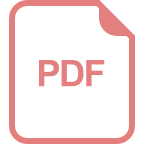














