定义一个合数世纪为一个世纪中所有100个年号都不是素数(即都是合数)。使用枚举法找出最早的合数世纪。用C语言编写程序
时间: 2024-10-18 07:19:59 浏览: 30
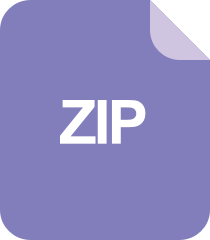
数学判断一个数是否为素数
在C语言中,我们可以创建一个枚举结构来表示各个年份,并使用循环判断每个年份是否为素数。然后,遍历从公元1到999年(因为1000年是一个新的千禧年),如果找到连续的100个非素数年份,就找到了最早的合数世纪。以下是简单的C代码实现:
```c
#include <stdio.h>
#include <stdbool.h>
// 判断是否为素数
bool is_prime(int num) {
if (num <= 1) return false;
for (int i = 2; i * i <= num; i++) {
if (num % i == 0)
return false;
}
return true;
}
// 枚举年份并查找合数世纪
enum Century { NOT_FOUND, CURRENT_CENTURY } find_first_composite_century(int year);
enum Century check_year(int year) {
// 如果当前年份不是素数,则返回当前世纪
if (!is_prime(year)) return CURRENT_CENTURY;
// 否则检查下一个年份,直到找到连续的非素数年份
return year + 1 == 100 ? NOT_FOUND : check_year(year + 1);
}
enum Century find_first_composite_century(int year) {
enum Century century_status = NOT_FOUND;
while (true) {
// 遍历当前世纪
int start_of_century = year - year % 100 + 1;
int end_of_century = year;
if (end_of_century >= 100 && !is_prime(end_of_century)) {
century_status = CURRENT_CENTURY;
// 检查是否已经连续100个非素数
bool consecutive_composites = true;
for (int i = start_of_century; i <= end_of_century; i++) {
if (is_prime(i))
consecutive_composites = false;
if (!consecutive_composites && i - start_of_century + 1 == 100)
break;
}
if (consecutive_composites)
return century_status;
}
year += 100;
}
}
int main() {
int start_year = 1; // 或者从公元1年开始
enumCentury result = find_first_composite_century(start_year);
if (result == CURRENT_CENTURY) {
printf("最早的合数世纪开始于%d年\n", start_year);
} else {
printf("找不到最早的合数世纪\n");
}
return 0;
}
```
阅读全文
相关推荐
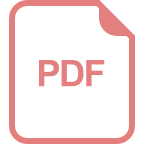
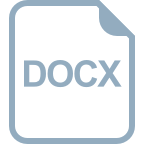
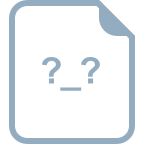
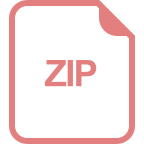









