用cpp语言制作一个程序,要求写出完整的代码,该程序要求实现散列表中的查找和插入功能.
时间: 2023-02-05 17:59:31 浏览: 54
首先,你需要创建一个散列表结构体,它应该包含一个数组和一个散列函数。数组用于存储数据,散列函数用于计算数据的散列值。
在这里,我们使用模数运算来实现散列函数。
struct HashTable {
// 散列表的大小
int size;
// 数组用于存储数据
int* data;
// 散列函数
int hash(int key) {
return key % size;
}
};
接下来,你需要写一个函数来查找数据。这个函数需要使用散列表和要查找的数据的键来计算数据的位置,并在散列表的数组中查找它。如果找到了数据,则返回数据的值;如果没有找到,则返回-1。
int find(HashTable* ht, int key) {
// 计算数据的位置
int index = ht->hash(key);
// 在散列表的数组中查找数据
int value = ht->data[index];
// 如果找到了数据,则返回数据的值
if (value != -1) {
return value;
}
// 如果没有找到,则返回-1
return -1;
}
最后,你需要写一个函数来插入数据。这个函数需要使用散列表和要插入的数据的键来计算数据的位置,并在散列表的数组中插入数据。
void insert(HashTable
相关问题
用cpp语言制作一个程序,要求写出完整的代码,该程序要求实现散列表中的查找和插入功能.
我们可以先定义一个结构体来表示散列表中的每一个节点,其中包含一个键值和一个值,然后再定义一个结构体来表示散列表本身,其中包含一个桶的数组和一个表示散列表大小的变量。
下面是实现查找和插入功能的代码:
```cpp
#include <iostream>
#include <unordered_map>
using namespace std;
struct Node {
int key;
int value;
Node *next;
Node(int k, int v) : key(k), value(v), next(NULL) {}
};
struct HashTable {
int size;
Node **bucket;
HashTable(int s) : size(s) {
bucket = new Node*[size];
for (int i = 0; i < size; i++) {
bucket[i] = NULL;
}
}
int hash(int key) {
return key % size;
}
void insert(int key, int value) {
int index = hash(key);
Node *node = new Node(key, value);
node->next = bucket[index];
bucket[index] = node;
}
Node *search(int key) {
int index = hash(key);
Node *node = bucket[index];
while (node != NULL) {
if (node->key == key) {
return node;
}
node = node->next;
}
return NULL;
}
};
int main() {
HashTable ht(10);
ht.insert(1, 10);
ht.insert(2, 20);
ht.insert(3, 30);
Node *node = ht.search(2);
if (node == NULL) {
cout << "Key not found" << endl;
} else {
cout << "Key found: " << node->value << endl;
}
return 0;
}
```
请注意,上面的代码使用的是链地址法来解决散列冲突的问题,但也可以使用其他的解决方案,例如开放地址法。
用cpp语言制作一个程序,要求写出完整的代码,该程序要求用桶的散列来实现散列表中的查找和插入功能.
首先, 您需要了解如何在 C++ 中实现散列表和桶。散列表是一种用于快速查找和插入数据的数据结构。桶是散列表中的一个数组, 它用于存储数据。
在实现散列表的查找和插入功能之前, 您需要了解如何计算散列值。散列值是用于将数据映射到桶中的一个数字。一种常见的方法是使用哈希函数。哈希函数接受数据作为输入, 并返回一个散列值。
下面是一个使用桶的散列表实现散列表查找和插入功能的例子:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义散列表类
class HashTable {
private:
// 桶的数量
int bucket_count;
// 桶的 vector
vector<int> buckets;
public:
// 构造函数
HashTable(int count) {
bucket_count = count;
// 初始化桶
for (int i = 0; i < bucket_count; i++) {
buckets.push_back(-1);
}
}
// 计算散列值的函数
int hash(int key) {
return key % bucket_count;
}
// 查找数据的函数
int find(int key) {
// 计算散列值
int h = hash(key);
// 在桶中查找数据
return buckets[h];
}
// 插入数据的函数
void insert(int key) {
// 计算散列值
int h = hash(key);
// 将数据插入桶中
buckets[h] = key;
}
};
int main() {
// 创建散列表,
相关推荐
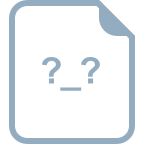












