l1.next = self.mergeTwoLists(l1.next, l2)
时间: 2023-12-15 22:04:14 浏览: 74
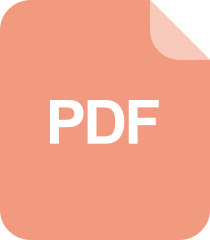
基于L1-L2规范的视觉跟踪目标表示
这段代码是一个递归调用,用于合并两个链表。其中,l1和l2分别是两个链表的头节点,mergeTwoLists函数的作用是将两个链表合并成一个有序链表,并返回合并后的链表头节点。
具体而言,这段代码的含义是:将l1和l2中头节点较小的一个节点作为新链表的头节点,然后递归调用mergeTwoLists函数,将剩余的节点继续合并。如果其中一个链表已经为空,则直接将另一个链表接到新链表的末尾即可。最终,返回合并后的链表的头节点。
需要注意的是,这段代码的前提条件是两个链表已经是有序的。
阅读全文
相关推荐
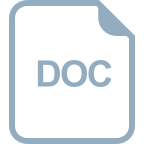
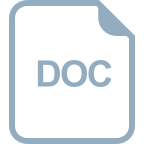















