python 把{'add':'123','bcs':'456'}的用AES256_GCM加密
时间: 2024-01-26 19:03:02 浏览: 245
你可以使用Python中的cryptography库来实现AES256_GCM加密。以下是一个例子:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.primitives.asymmetric import padding
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives import serialization
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
from cryptography.hazmat.primitives import padding as symmetric_padding
import base64
def encrypt_aes_gcm(data, key):
# 生成随机的12字节IV(Initialization Vector)
iv = os.urandom(12)
# 创建AES-GCM加密算法实例
cipher = Cipher(algorithms.AES(key), modes.GCM(iv), backend=default_backend())
# 创建加密器
encryptor = cipher.encryptor()
# 加密数据
ciphertext = encryptor.update(data) + encryptor.finalize()
# 获取加密后的认证标签(tag)
tag = encryptor.tag
# 返回加密后的密文、IV和tag
return ciphertext, iv, tag
def main():
# 待加密的数据
data = b"{'add':'123','bcs':'456'}"
# 密钥(32字节)
key = b'your_key_in_bytes'
# 使用AES256-GCM加密数据
ciphertext, iv, tag = encrypt_aes_gcm(data, key)
# 将密文、IV和tag转换为Base64编码的字符串
ciphertext_b64 = base64.b64encode(ciphertext).decode('utf-8')
iv_b64 = base64.b64encode(iv).decode('utf-8')
tag_b64 = base64.b64encode(tag).decode('utf-8')
print("Ciphertext:", ciphertext_b64)
print("IV:", iv_b64)
print("Tag:", tag_b64)
if __name__ == '__main__':
main()
```
请注意,上述代码中的`your_key_in_bytes`需要替换为您自己的密钥。此外,为了运行此示例,您需要安装cryptography库。可以使用以下命令安装:
```
pip install cryptography
```
运行上述代码后,您将获得AES256-GCM加密后的密文(ciphertext)、初始化向量(IV)和认证标签(tag)。
阅读全文
相关推荐
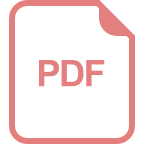
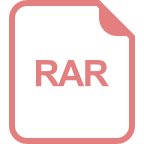
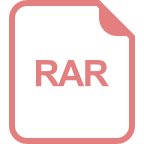
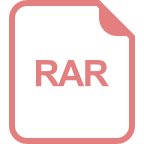
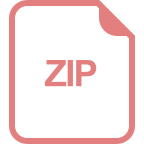
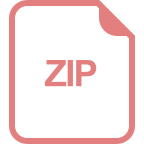
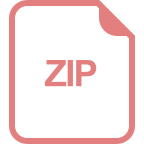
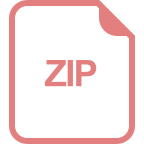
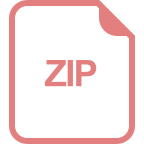
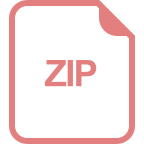
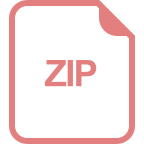
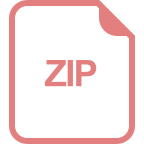
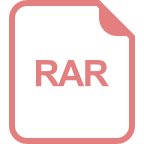