写出分治法快速排序 的c语言程序
时间: 2024-10-22 13:02:41 浏览: 26
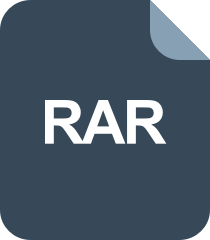
C语言算法源代码
分治法是一种常见的算法设计策略,用于解决可以分解成若干个规模较小的相同问题的问题。在快速排序(Quick Sort)中,这个过程通常涉及选择一个基准元素、分区操作和递归调用自身。以下是一个简单的C语言实现:
```c
#include <stdio.h>
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
int partition (int arr[], int low, int high) {
int pivot = arr[high]; // 基准元素
int i = (low - 1); // Index of smaller element
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++; // increment index of smaller element
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]); // Swap pivot with the correct position
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
/* pi is partitioning index, arr[p] is now
at right place */
int pi = partition(arr, low, high);
// Separately sort elements before
// partition and after partition
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
// 主函数测试
int main() {
int data[] = {9, 7, 5, 11, 12, 2, 14, 3, 10, 6};
int n = sizeof(data) / sizeof(data[0]);
printf("Original array: \n");
for (int i=0; i < n; i++)
printf("%d ", data[i]);
quickSort(data, 0, n-1);
printf("\nSorted array: \n");
for (int i=0; i < n; i++)
printf("%d ", data[i]);
return 0;
}
```
在这个程序中,`quickSort`函数是主功能,它首先检查低索引和高索引是否小于等于高索引。如果是,则调用`partition`函数将数组分成两部分,并递归地对这两部分进行排序。
阅读全文
相关推荐
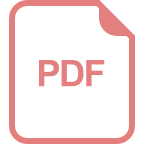
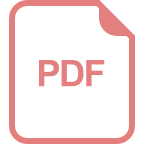

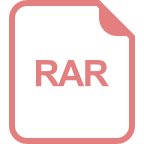
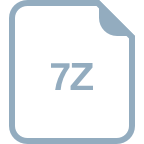
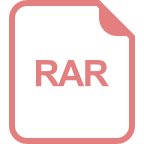
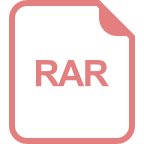
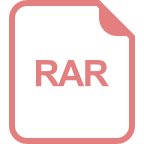
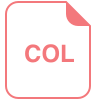
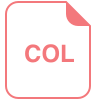







