作业调度算法JAVA实现
时间: 2024-10-24 16:06:34 浏览: 23
在Java中实现作业(任务)调度算法通常涉及操作系统原理和并发编程的知识。这里举一个简单的例子,比如常用的FIFO(先进先出)调度算法,可以使用Java中的`java.util.PriorityQueue`队列来模拟:
```java
import java.util.PriorityQueue;
public class JobScheduler {
private PriorityQueue<Job> schedule = new PriorityQueue<>((a, b) -> a.getPriority() - b.getPriority());
// 假设Job类有任务ID(priority)和执行时间(executionTime)
public static class Job implements Comparable<Job> {
int priority;
long executionTime;
// 构造函数、getters和setters省略
@Override
public int compareTo(Job other) {
return Integer.compare(this.priority, other.priority);
}
}
public void addJob(Job job) {
schedule.offer(job);
}
public void executeNextJob() {
if (!schedule.isEmpty()) {
Job nextJob = schedule.poll();
System.out.println("Executing job with ID " + nextJob.getId());
// 这里假设job的执行需要同步处理,所以在这里执行其业务逻辑
// nextJob.execute(); // 你需要自己实现这个方法
} else {
System.out.println("No more jobs to execute");
}
}
}
```
在这个例子中,我们创建了一个`JobScheduler`类,它维护了一个按照优先级排序的任务队列。通过`addJob`方法添加任务,`executeNextJob`方法会选择并执行优先级最高的任务。
阅读全文
相关推荐
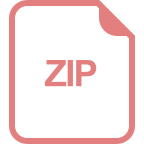
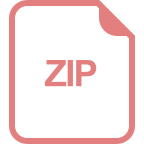
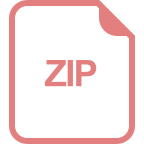
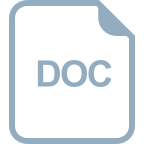
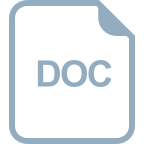
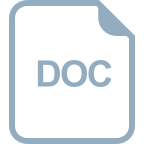
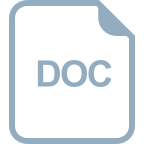



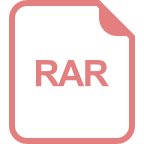
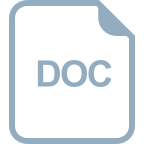
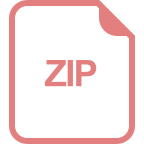
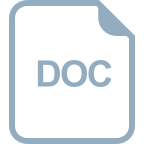
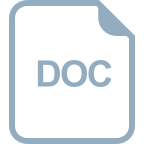
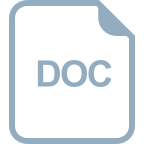

