用python并且调用pygame库写一个人机对战的五子棋游戏
时间: 2024-04-14 11:26:20 浏览: 168
当然可以!下面是一个使用Python和Pygame库编写的人机对战的五子棋游戏的示例代码:
```python
import pygame
import sys
import random
# 定义棋盘大小和格子大小
BOARD_SIZE = 15
GRID_SIZE = 40
# 定义窗口大小
WINDOW_WIDTH = BOARD_SIZE * GRID_SIZE
WINDOW_HEIGHT = BOARD_SIZE * GRID_SIZE
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (200, 200, 200)
# 初始化Pygame
pygame.init()
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("人机对战五子棋")
# 初始化棋盘
board = [['+' for _ in range(BOARD_SIZE)] for _ in range(BOARD_SIZE)]
# 当前下棋方,默认玩家先行
current_player = BLACK
# 判断是否胜利
def check_win(row, col):
directions = [(0, 1), (1, 0), (1, 1), (-1, 1)]
for dx, dy in directions:
count = 1
x, y = row, col
while count < 5:
x += dx
y += dy
if x < 0 or x >= BOARD_SIZE or y < 0 or y >= BOARD_SIZE or board[x][y] != current_player:
break
count += 1
x, y = row, col
while count < 5:
x -= dx
y -= dy
if x < 0 or x >= BOARD_SIZE or y < 0 or y >= BOARD_SIZE or board[x][y] != current_player:
break
count += 1
if count >= 5:
return True
return False
# 绘制棋盘
def draw_board():
window.fill(GRAY)
for i in range(BOARD_SIZE):
pygame.draw.line(window, BLACK, (GRID_SIZE // 2, i * GRID_SIZE + GRID_SIZE // 2),
(WINDOW_WIDTH - GRID_SIZE // 2, i * GRID_SIZE + GRID_SIZE // 2))
pygame.draw.line(window, BLACK, (i * GRID_SIZE + GRID_SIZE // 2, GRID_SIZE // 2),
(i * GRID_SIZE + GRID_SIZE // 2, WINDOW_HEIGHT - GRID_SIZE // 2))
# 绘制棋子
def draw_piece(row, col):
color = BLACK if board[row][col] == BLACK else WHITE
pygame.draw.circle(window, color, ((col + 1) * GRID_SIZE, (row + 1) * GRID_SIZE), GRID_SIZE // 2 - 2)
# 机器人玩家下棋
def robot_player_move():
valid_moves = get_valid_moves()
row, col = random.choice(valid_moves)
make_move(row, col)
draw_piece(row, col)
# 获取有效的下棋位置
def get_valid_moves():
valid_moves = []
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == '+':
valid_moves.append((i, j))
return valid_moves
# 下棋
def make_move(row, col):
if board[row][col] == '+':
board[row][col] = current_player
return True
return False
# 处理鼠标点击事件
def handle_mouse_click(pos):
if current_player == BLACK:
col = pos[0] // GRID_SIZE - 1
row = pos[1] // GRID_SIZE - 1
if row >= 0 and row < BOARD_SIZE and col >= 0 and col < BOARD_SIZE:
if make_move(row, col):
draw_piece(row, col)
if check_win(row, col):
show_message("黑方获胜!")
else:
current_player = WHITE
robot_player_move()
# 显示游戏结束信息
def show_message(message):
font = pygame.font.SysFont(None, 48)
text = font.render(message, True, BLACK)
text_rect = text.get_rect(center=(WINDOW_WIDTH // 2, WINDOW_HEIGHT // 2))
window.blit(text, text_rect)
pygame.display.update()
pygame.time.wait(3000)
pygame.quit()
sys.exit()
# 游戏循环
def game_loop():
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
handle_mouse_click(pygame.mouse.get_pos())
pygame.display.update()
# 运行游戏
draw_board()
game_loop()
```
这是一个使用Pygame库编写的人机对战的五子棋游戏,它提供了一个可视化的游戏界面。玩家可以使用鼠标点击下棋,机器人玩家会随机选择一个有效的下棋位置进行下棋。程序会判断是否有玩家获胜,并在游戏结束时显示相应的消息。希望对你有帮助!
阅读全文
相关推荐
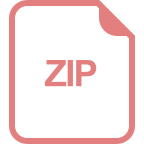

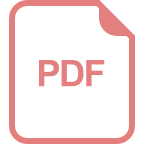


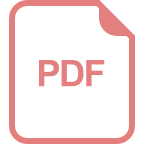
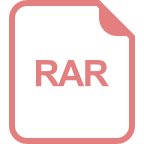
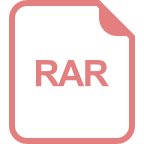
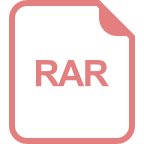
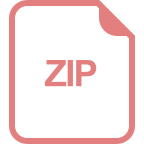
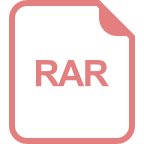
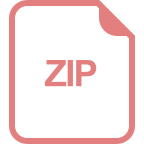
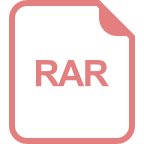
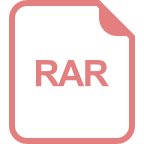
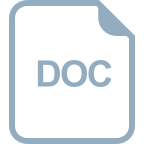
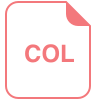
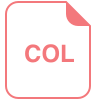